Web Programming
SOEN 287: Web Programming – Winter 2024 Assignment 2 ______________________________________________________________________________ Due Date: By 11:55pm Mar 29 (Friday), 2024 Evaluation: 7% of final mark Late Submission: none accepted Type: Individual Assignment Purpose: The purpose of this assignment is to have you practice JavaScript and use JavaScript to operate on DOM model CEAB Attributes: This assignments is primarily evaluating your use of JavaScript and DOM models hence use of engineering tools as well as design. ______________________________________________________________________________ Common Requirements • • All the code should pass the HTML validator (https://validator.w3.org/nu/#file) without any error or warning messages. All the code should have no error or warning message in the browser console after execution. Exercise 1 : Use JavaScript to Change Content of HTML Tag Figure 1 is the original HTML page. Figure 2 shows the page after clicking the button. The line above the button is changed. Requirements: • • • Define a function (e.g. changeit()) as the event handler in the head of the html Register the event handler to the button (e.g. Click the button, the content of a is changed to “Paragraph changed” Figure 1: the original html page Figure 2: the changed html page SOEN287/Fall 2024 Assignment #2-Page 1 Exercise 2: Print JavaScript Array In this exercise, you can put javascript code in inside tag. First, use const to define an array, e.g. const arryStr = const fruits = [“Banana”, “Orange”, “Apple”, “Mango”]; You can mix the other datatype in the array. Then implement the follow functions. A. Print all the elements of the array using toString() and insert the output string inside a tag. B. Print all the elements of the array using join() and separate the elements using “&”. Inset the output string inside a tag. C. Print the array in the format of index: value. For example: 0: Banana 1: Orange 2: Apple 3: Mango You can use Array.foreach() or your own implementation The results are demoed in Figure 3. Figure 3: Print the elements in an array of Strings SOEN287/Fall 2024 Assignment #2-Page 2 Exercise 3: JavaScript functions All your JavaScript functions must be declared in an external .js file which is linked to an HTML file. Each functions name must be as specified below. To demonstrate the functionality of each method, you must make function calls in the document body and insert the results in a html tag. Please use const for array declaration and use let for normal variables. Avoid using global variables. A. Function: addNumbers Parameter(s): Array of numbers Each element in the array must be added and the summation (answer) must be returned. You can either implement it using loop or call array.reduce(). B. Function: findMaxNumber Parameter(s): None (Hint: Make use of the arguments array) From the arguments array, find the number element that is the largest and return it. You can either implement it using loop or call Math.max(). C. Function: addOnlyNumbers Parameter(s): Array of mixed data type Convert all other datatypes into number using parseFloat(). Please notice parseFloat(“3 birds”) = 3. So addOnlyNumbers([4, 5, “3.0 birds”, true, “birds2″])=12. D. Function: getDigits Parameter(s): A String Scan the string and find all the digits (0-9), concatenate them into a string in the order that they are found and return the string of numbers. Use patterns. E. Function: reverseString Parameter(s): A String Reverse the entire string (character by character) and return the resulting string. For example, reverseString(“See you later”) returns “!retal uoy eeS” F. Function: getCurrentDate Parameter(s): None Retrieve the current date in the format similar to: Sunday, Feb 19, 2023 and return it. Use Date. SOEN287/Fall 2024 Assignment #2-Page 3 Exercise 4: Calculate your order Please reference to the demo implementation below. First, design an online order page, with several items and their prices (you can give any items and prices). Allow the visitor to enter the quantity of each item. After the visitor presses “Place order” button, the total cost is displayed on the same page beneath the form. All JavaScript code must be external. If any fields are left blank or do not contain an integer (must be an integer), an alert box should display an appropriate error message upon form submission. Hint: use pattern matching. Figure 4: Before the “Place Order” button is pressed Figure 5: After the “Place Order” button is pressed SOEN287/Fall 2024 Assignment #2-Page 4 Exercise 5: Pop up Windows Given the following HTML page: Exercise 5 User Information: Create an embedded JavaScript function named getUserInfo that prompts the user with the following two questions after the HTML page has loaded: • • What is your full name? How old are you? The function should then build a string in the form of: Hi, my name is NAME and I’m XX years old. The string must then be inserted into the container div with id: content. SOEN287/Fall 2024 Assignment #2-Page 5 Exercise 6: A simple slide show Slide show is normally implemented by JavaScript. Here is a simple one. This page shows a news about gravitational wave discovery. Under the image, there are two links. When you click the link, the image changes to the next image. Please implement this slide show. The text and the images used for this exercise are from a BBC news report on Feb 11, 2022. The link http://www.bbc.com/news/science-environment-35523676. Just for classroom use. Figure 6: Slide 1 Figure 6: Slide 2 SOEN287/Fall 2024 Assignment #2-Page 6 Figure 6: Slide 3 Figure 6: Slide 4 SOEN287/Fall 2024 Assignment #2-Page 7 Exercise 7: Step 2 of the Running Project – Adopt a Dog/Cat Following are the additions for the Pet Adoption Website started in assignment 1 1) Date and Time: In the header area of your website, use JavaScript to display the current date and time with second precision. The Time should automatically refresh and show the updated time every second. 2) Browse Available Pets page: Create a Browse Available Pets page pets.html linked from the side menu. Inside the content area of this page, create a list of a couple of fictitious pets available for adoption.: pets.html. Include an image, along with the information found in the Have a pet to give away page. Make sure the format of the page is easy to read and that the look is consistent with the rest of your site. Next to each pet, add a button labeled “Interested”. In this iteration of the project, clicking on the button need not do anything. 3) Find a dog/cat page: The form was created in the previous assignment. In this assignment, you are required to implement client-side validation using JavaScript. Upon pressing the form’s submit button, ensure that no fields are left blank. If any fields are blank upon submitting the form, display an error message, but retain the rest of the form as the user had input before, without resetting the filled values. 4) Have a pet to give away page: The form was created in the previous assignment. In this assignment, you are required to implement client-side validation using JavaScript. Upon pressing the form’s submit button, ensure that no fields are left blank and that the provided email follows a valid format. If any fields are blank or invalid upon submitting the form, display an error message, but retain the rest of the form as the user had input before, without resetting the filled values. Hint: Regular Expressions are your friend, but you should look up what a valid format for an email is. Note on URL Paths Ensure that you consistently use “relative file paths” in your project when linking or loading JS, CSS, and image files in your HTML code. Avoid using “absolute file paths,” such as “C:UsersBobProjectsSOEN287A2Q2.CSS” or “http://localhost:1313/SOEN287/A2/Q2.CSS,” as they cause your project to malfunction on a different machine during marking. For example, the URL of the menu button linking to the “Find a Dog/Cat” page should be simply written as href=”find.html”, instead of using the absolute file path like href=”C:UsersBobProjectsSOEN287A2Q7find.html”. For more information, please refer to the following link: https://www.w3schools.com/html/html_filepaths.asp SOEN287/Fall 2024 Assignment #2-Page 8 Submitting Assignment 2 • • • • Place all HTML files for Q1 to Q6 in the root directory of your project, while their corresponding CSS and JS files can be organized in subdirectories as per your preference. As for Q7, place all files in a subdirectory named “Q7”. When submitting your assignment, create a single zip file that includes all folders and files required for the project. Please give meaningful names to files to make the evaluation process easier. The zip file should be called a#_studentID, where # is the number of the assignment and studentID is your student ID number. For example, for this second assignment, student 123456 would submit a zip file named a2_123456.zip Please make sure to review your final version right before submission and avoid making unreviewed last-minute changes. The submitted file will be graded, and it is your responsibility to ensure it is the correct version. Evaluation Criteria for Assignment 2 (26 points) Question 1 – 3 pts. • • • “changeit” Function Event Handler Correct Functionality 0.5 pts 0.5 pts 2 pts Question 2 – 3 pts. a) toString b) join c) index: value 1 pt 1 pt 1 pt Question 3 – 6 pts. a) b) c) d) e) addNumbers() findMaxNumber() addOnlyNumbers() getDigits() reverseString() f) getCurrentDate () 1 1 1 1 1 1 pt pt pt pt pt pt Question 4 – 4 pts. • Order Form Page SOEN287/Fall 2024 1 pt Assignment #2-Page 9 • • 1 pt 1 pt Make sure all fields have an integer Calculate & display total as well as summary of order Question 5 – 2 pts • • 1 pt 1 pt Prompt User for Inputs Display the Output String Question 6 – 2 pts • Implement simple slide show 2 pts Question 7 – 6 pts. • • • • 1 1 1.5 2.5 Date and time in header Browse available pets Validate input for Find a dog/cat page Validate input for Have a pet to give away page TOTAL SOEN287/Fall 2024 pt pt pts pts 26 pts Assignment #2-Page 10
Collepals.com Plagiarism Free Papers
Are you looking for custom essay writing service or even dissertation writing services? Just request for our write my paper service, and we'll match you with the best essay writer in your subject! With an exceptional team of professional academic experts in a wide range of subjects, we can guarantee you an unrivaled quality of custom-written papers.
Get ZERO PLAGIARISM, HUMAN WRITTEN ESSAYS
Why Hire Collepals.com writers to do your paper?
Quality- We are experienced and have access to ample research materials.
We write plagiarism Free Content
Confidential- We never share or sell your personal information to third parties.
Support-Chat with us today! We are always waiting to answer all your questions.
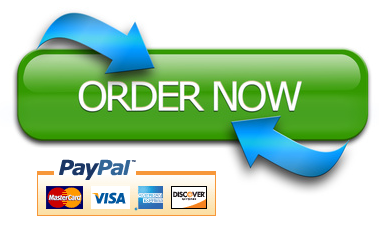