Word manipulation
Write a program that performs the following steps:
1. On start, prompt the user to enter a sentence, and let them type it in. This can be any numbers of words/characters/spaces, with the newline character (when the user presses the the enter key) indicating the end of the string.
2. Secondly, prompt the user for a WORD. This should be just a single word, no whitespace. You can assume the user will abide, here.
3. Based on the user’s entered sentence, and the user’s entered word, print out the following statistics regarding the sentence:
the number of punctuation characters in the sentence
the number of vowels in the sentence (including both upper and lowercase vowels).
the number of words in the sentence (counting spaces here can help.)
A word here is defined “as any set of any characters containing no whitespace.”
a table of word lengths (Note that our words will be from 1 to 20 characters long).
a message if the user entered WORD was part of the SENTENCE they entered or not. It must be an exact match (character case matters here). Note that for this task, you are NOT PERMITTED to use the string library function “find”. You need to build your own segment of code that checks to see if the user entered word is a substring (is contained within) of the sentence they entered.
4. Ask the user if they wish to enter another sentence/word pair and perform the task over again. They can enter the word “yes” or “no” here as their response, case here is irrelevant. So, consider any version of YES (“yes”,”YES”,”Yes”,”yES”…) as the same, and the same idea for “no”. Make sure not to allow a bad entry here — force the user to enter yes or no. You may assume this entry from the user will be only one word.
Information if you choose to use C-Strings:
You may assume the sentence string that the user enters will be no more than 100 characters long, so you can declare your array accordingly. You can also assume the maximum length of any word entered (whether a part of the sentence or just the standalone word) is 20 characters.
HINTS/ASSUMPTIONS/BONUS POINTS:
Insert the line:
cin.ignore();
After you read in the user’s yes/no entry if you’re having trouble reading in another sentence upon the user choosing “yes”.
YOU MAY ASSUME that the sentence the user enters will have only exactly one whitespace between each word.
If you want to account for sentences like such:
This is a test sentence there are nine words.
and have the valid # of words print out here in this case as well, I’ll award 5 bonus points if you accomplish this. Sample run 2 gives an example of this.
You may also assume that anytime the user is prompted to enter only one word, that they will do so.
When working on the word length table: we need to keep a counter for each word size! This means, we need TWENTY counter variables. One for the number of words of length 1, one for the number of words with length 2… so, it may be helpful to declare an array of integers for this task. Try declaring an array of integers of size 21. Initialize all elements of this array to be 0. You’ve effectively just created 21 counter variables. You can now use this array of counter variables to help keep track of the number of words of each length.
You can also use this array with the idea that: array[1] would store the number of words of size 1, array[2] would store the number of words of size 2, array[3] would store the number of words of size 3, … and so on.
With this thought, array index 0 just goes unused. You can declare the array of integers originally of size 20 instead of 21, but then be aware that the array index value would correspond to wordsize -1 instead of a 1 to 1 correspondence.
Functions:
YOU are the programmer now. So, decide which functions you’d like to build to complete your program.
The only rule is that you must have at least two (2) functions in addition to main() that serve a VALID USE in your program.
You can name your functions whatever you’d like.
It’s also up to YOU to figure out what parameters the functions you write should take in, and what you’d like them to return, if anything.
You’re welcome to write any additional functions (i.e. more than 2) to complete the required tasks in your program.
I’d recommend reading in the sentence and the word from the user in main(), and then passing those pieces of data off to some functions you build that use them.
Recommended ideas for functions:
A function that takes in the sentence and the word and returns true or false based on if the word is in the string or not
A function that takes in the sentence and returns the number of punctuation characters in it
A function that takes in the sentence and returns the number of vowels in it
A function that takes in the sentence and returns the number of words in it
A function that takes in the sentence and prints out the word length table
A function that takes in a string and changes it to all upper or all lowercase (this can help with Yes yES yEs yeS …)
… or come up with some different ideas or variations of the above!
Sample Run 1:
Enter a sentence: When in doubt, pinky out!
Enter a word: PINKY
SENTENCE ANALYSIS:
Punctuation Characters: 2
Vowels: 7
Words: 5
Word Lengths:
2 characters long: 1 word.
4 characters long: 2 words.
5 characters long: 1 word.
6 characters long: 1 word.
The word: “PINKY” IS NOT part of the sentence you entered.
Enter another sentence/word for analysis (yes/no): YEs
Enter a sentence: Licking doorknobs is illegal on other planets.
Enter a word: planet
SENTENCE ANALYSIS:
Punctuation Characters: 1
Vowels: 14
Words: 7
Word Lengths:
2 characters long: 2 words.
5 characters long: 1 word.
7 characters long: 2 words.
8 characters long: 1 word.
9 characters long: 1 word.
The word: “planet” IS a part of the sentence you entered.
Enter another sentence/word for analysis (yes/no): blah
Enter yes or no: yeet
Enter yes or no: yadayada
Enter yes or no: yes
Enter a sentence: 1234 567 89
Enter a word: boo
SENTENCE ANALYSIS:
Punctuation Characters: 0
Vowels: 0
Words: 3
Word Lengths:
2 characters long: 1 word.
3 characters long: 1 word.
4 characters long: 1 word.
The word: “boo” IS NOT part of the sentence you entered.
Enter another sentence/word for analysis (yes/no): no
Sample Run 2: (Illustrating extra credit option)
Enter a sentence: Extra credit the teacher’s ace in the hole
Enter a word: ache
SENTENCE ANALYSIS:
Punctuation Characters: 1
Vowels: 14
Words: 8
Word Lengths:
2 characters long: 1 word.
3 characters long: 3 words.
4 characters long: 1 word.
5 characters long: 1 word.
6 characters long: 1 word.
9 characters long: 1 word.
The word: “ache” IS a part of the sentence you entered.
Enter another sentence/word for analysis (yes/no): no
Requirements:
No global variables
Only one return statement is permitted in main(). The one at the end.
You may use any of these libraries:
iostream
iomanip
cctype
cstring
Collepals.com Plagiarism Free Papers
Are you looking for custom essay writing service or even dissertation writing services? Just request for our write my paper service, and we'll match you with the best essay writer in your subject! With an exceptional team of professional academic experts in a wide range of subjects, we can guarantee you an unrivaled quality of custom-written papers.
Get ZERO PLAGIARISM, HUMAN WRITTEN ESSAYS
Why Hire Collepals.com writers to do your paper?
Quality- We are experienced and have access to ample research materials.
We write plagiarism Free Content
Confidential- We never share or sell your personal information to third parties.
Support-Chat with us today! We are always waiting to answer all your questions.
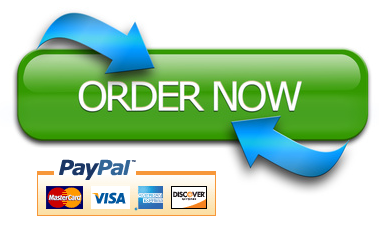