you are on the testing team at work. unfortunately, your company’s programmers do not have good testing practices.
you are on the testing team at work. unfortunately, your company’s programmers do not have good testing practices. there aren’t many tests written, and the ones that are out there don’t work reliably: they pass some of the time, but they often fail for unrelated reasons. to force your developers get their testing act together, your boss wants you to write a tool to run a test multiple times and only pass if the tests passes all of the times. you have to write a flake program that will rerun a test until it fails or passes all of the tries. passing means that it exit with a 0 exit code. flake will keep track of stdout, stderr, and the exit code of the program. if the flake is able to run the program successfully all of the tries, it will print the stdout of the last successful run to stdout, the stderr of the last successful run to stderr, and exit with exit code of 0. Otherwise, it will output the stdout and stderr of the last run of the program and exit with the exit code of that run.
if the test program dies due to a signal, use 100+ signal_number as the exit code.
the flaky tests sometimes hang, so if a timeout is exceeded, you need to stop the program with a SIGKILL.
you will use execvp to execute the program. if execvp fails, use perror passing the name of the test_command and exit with a code of 2.
*SPECIFICATION *
flake max_tries max_timeout test_command args…
max_tries – is the maximum amount of times you will try rerunning the test before declaring success.
max_timeout – the maximum allowed time for a test to run
test_command – the command you will be running for the test
args – any arguments the test may take
you will need to create temporary files to track output. make sure they are deleted before flake exits.
* USAGE *
$ ./flake
USAGE: ./flake max_tries max_timeout test_command args…
max_tries – must be greater than or equal to 1
max_timeout – number of seconds greater than or equal to 1
$ echo $?
1
$ ./flake not_num not_num cmd asdf
USAGE: ./flake max_tries max_timeout test_command args…
max_tries – must be greater than or equal to 1
max_timeout – number of seconds greater than or equal to 1
$ echo $?
1
* CALLS TO USE *
when you implement flake, you will need to use the following system calls: fork, execvp, wait, open, dup2, signal, kill, and alarm.
to get the exit codes from that status returned from wait please look at the macros (they act like functions) in the man page for wait (man 2 wait). when you open a file with create, you will need to set the mode, so you will probably invoke it like:
open(name, O_RDWR | O_CREATI O_TRUNC, S_IRUSR | S_IWUSR)
* Compiling Your Code *
gcc -g -std=gnu2x -Wall -o flake flake.c
* EXAMPLES *
Command to RUN: ./runvalgrind.sh ./flake
Expected Exit Code: 1
USAGE: ./flake max_tries max_timeout test_command args…
max_tries – must be greater than or equal to 1.
max_timeout – number of seconds greater than or equal to 1.
Command to RUN: ./runvalgrind.sh ./flake 1 1 echo hi
Expected Exit Code: 0
hi
Command to RUN: /runvalgrind.sh ./flake 1 1 badcmd the command is invalid
Expected Exit Code: 2
badcmd: No such file or directory
Command to RUN: ./runvalgrind.sh ./flake 3 3 sleep 1
Expected Exit Code: 0
Command to RUN: ./flake 1 1 sleep 2
Expected Exit Code: 109
Collepals.com Plagiarism Free Papers
Are you looking for custom essay writing service or even dissertation writing services? Just request for our write my paper service, and we'll match you with the best essay writer in your subject! With an exceptional team of professional academic experts in a wide range of subjects, we can guarantee you an unrivaled quality of custom-written papers.
Get ZERO PLAGIARISM, HUMAN WRITTEN ESSAYS
Why Hire Collepals.com writers to do your paper?
Quality- We are experienced and have access to ample research materials.
We write plagiarism Free Content
Confidential- We never share or sell your personal information to third parties.
Support-Chat with us today! We are always waiting to answer all your questions.
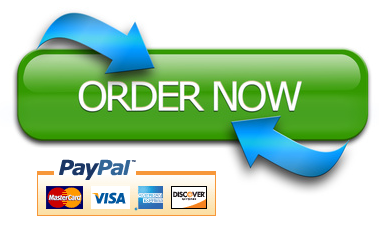