The purpose of this assignment is to get you started writing simple lines of code in JavaScript. It will be done making something artistic – not always the end use case of code, but coding
The purpose of this assignment is to get you started writing simple lines of code in JavaScript. It will be done making something artistic – not always the end use case of code, but coding can be a way to express yourself! This assignment will have you writing commands, or lines of code that do something, and variables, which are lines of code that help you create and store data. As a first assignment, this is deceptively easy. Future assignments will require more effort and time.
It is expected that you do this work without referencing material beyond those employed in the class. (i.e., not using stack overflow or other resources to find exact solutions to the problems posed).
In this assignment and each future assignment you will be asked to create an algorithm (or plan) for your code, which will be shown in your comments in the code itself. You may also wind up doing some of your thinking on paper before turning it into a plan you can create code for.
Additionally, you will be required to write a brief reflection on your experience.
Submit a word document that includes :
1-your code
2- A reflection on the process you employed to get to this outcome (how did you plan your code, what resources did you use, where did you struggle, what were your takeaways / insights?)
3-The planning material you used for your program (pseudo code, algorithm development, drawings/sketches/flowcharts)
View Rubric
Lab01/index.html
Home || List Books
Lab01/www/img/books/civil_war.jpg
Lab01/www/img/books/greater_journey.jpg
Lab01/www/img/bookstore.gif
,
I210 Information Infrastructure I
Copyright © Louie Zhu 1
Unit 01: HTML Fundamentals
Lab 01: Creating Webpages for PHP Online Bookstore
In this lab, you will build a simple Web site containing four Web pages. Since HTML is a client-side
language, live demo of the site is not available before the due date of the lab. Once the lab is due, you
can view the live demo and solution code at https://i210.sitehost.iu.edu/index.php?u=1. The following
screen shots show the four pages you will need to complete in this lab.
To make the site look consistent, a common navigation bar and a banner appear on every page. The
diagram below shows the layout of every page on the site. It starts with a div block for the navigation
bar, which is followed by a table with one row and two columns. After the table, there is another div
civilwarbattlefieldguide.html greaterjourney.html
index.html book.html
I210 Information Infrastructure I
Copyright © Louie Zhu 2
body for the main content of a page. This content is page specific and varies from page to page. The
layout ends with another div block of footer.
Navigation bar containing two links: Home and List Books
Logo image Banner text
Main body
Footer with copyright information
Getting Ready
1. Download the Lab01.zip file from Canvas. Extract it into the htdocs/I210 folder on your
computer. The extraction should create a folder named Lab01 with one subfolder named www
and one file named index.html. Inside the www folder, there are image files. Carefully exam the
folder hierarchy and the existing code in the index.html file.
Modifying the index.html file
2. Start PhpStorm and open the I210 project. Open index.html in Lab01 folder.
3. Locate the div block for the navigation bar. It is clearly labeled with html comments.
4. Turn the word “Home” into a hyperlink that points to “index.html” file.
5. Turn the phrase “List Books” into a hyperlink that points to “books.html”.
6. Locate the table for the logo image and banner text. The table contains one row and two cells.
7. Insert the bookstore.gif image into the left cell. Use the width and align attributes to set its
width and alignment as follows:
<img src="www/img/bookstore.gif" width="200" alt="PHP Online Bookstore" align="left"/>
8. In the second cell, create h1 and h3 headings for the banner text.
<h1>PHP Online Bookstore</h1>
<h3>Learn how to build an online bookstore<br>with PHP and MySQL</h3>
I210 Information Infrastructure I
Copyright © Louie Zhu 3
9. Inside the div block of the main body of content, create an h2 heading.
<h2>Welcome to PHP Online Bookstore</h2>
10. Add two paragraphs below the h2 heading.
<p>This web site demonstrates a simple shopping cart application. It uses sessions to
maintain states. Click links at the top navigation bar to try different features. The
shopping cart content can be displayed by clicking the link at the upper-right
corner.</p>
<p>Major features include:</p>
11. Following the paragraph, create an unordered list.
<ul> <li>List books</li>
<li>Search books with keywords in book titles</li>
<li>Login/logout</li>
<li>Register/create new accounts</li>
<li>Add new books (administrators only)</li>
</ul>
12. Inside the div block of the footer, add a horizontal rule and a paragraph for the copyright
information.
<hr>
<p> © PHP Online Bookstore. All Rights Reserved.</p>
13. You have completed the index.html file. Save the file and view it in a browser. Make the page
appears the same as the screenshot on the previous page.
Creating the books.html file
14. Copy index.html file in PhpStorm and paste it in the Lab01 folder. Name the file as books.html.
15. Open books.html in PhpStorm. In the next few steps, you will modify the content in the main
body section of the list books Web page. You should not need to change anything else in the file.
16. Change the text of the h2 heading to “Books in Our Store”.
17. Delete the two paragraphs and the unordered list below the h2 heading.
18. Below the h2 heading, insert a new table using following settings:
a. Rows: 6
b. Columns: 4
c. Width: 800
d. Border width: 1
e. Cell spacing: 0
f. Cell padding: 5
19. Format the first row into table headings.
I210 Information Infrastructure I
Copyright © Louie Zhu 4
20. Fill the table with following content:
21. Turn the book title "The Civil War Battlefield Guide" into a hyperlink to
"civilwarbattlefieldguide.html".
22. Turn the book title "The Greater Journey: American in Paris" into a hyperlink to
"greaterjourney.html".
23. View the file in a browser. Make sure the two hyperlinks in the navigation bar work.
Creating the civilwarbattlefieldguide.html file
24. Create a new HTML file in PhpStorm and save it as civilwarbattlefieldguide.html into the Lab01
folder. Delete all the code in the file.
25. Copy all the code in the index.html file and paste it into the civilwarbattlefieldguide.html file.
26. Locate the div block of the main body of content. Change the text of the h2 heading to "The Civil
War Battlefield Guide".
27. Delete the two paragraphs and the unordered list below the h2 heading.
28. Below the h2 heading, insert a new table with the following settings:
a. Rows: 7
b. Columns: 3
c. Border width: 0
d. Cell spacing: 0
e. Cell padding: 5
f. Widths of the three columns: 200, 65, and 300 pixels.
29. Merge all seven cells in the first column into one spanning cell. Insert the book image into the
spanning cell using following settings:
a. Image file: civil_war.jpg
b. Location: www/img/books
c. Image width: 150pixels
d. Alternative text: The Civil War Battlefield Guide
30. Fill the second and third columns of the table with the following content:
I210 Information Infrastructure I
Copyright © Louie Zhu 5
31. Below the table, create a paragraph and a hyperlink to books.html.
32. View the file in a browser.
Creating the greaterjourney.html file
33. Create a new HTML file in PhpStorm and save it as greaterjourney.html into the Lab01 folder.
Delete all the code in the file.
34. Copy all the code in the index.html file and paste it into the greaterjourney.html file.
35. Locate the div block of the main body of content. Change the text of the h2 heading to "The
Greater Journey: Americans in Paris".
36. Delete the two paragraphs and the unordered list below the h2 heading.
37. Below the h2 heading, insert a new table with the following settings:
a. Rows: 7
b. Columns: 3
c. Border width: 0
d. Cell spacing: 0
e. Cell padding: 5
f. Widths of the three columns: 200, 65, and 300 pixels.
38. Merge all seven cells in the first column into one spanning cell. Insert the book image into the
spanning cell using following settings:
a. Image file: greater_journey.jpg
b. Location: www/img/books
c. Image width: 150 pixels
d. Alternative text: The Greater Journey: Americans in Paris
39. Fill the second and third columns of the table with the following content:
I210 Information Infrastructure I
Copyright © Louie Zhu 6
40. Below the table, create a paragraph and a hyperlink to books.html.
41. You have completed all html files. Open index.html in a browser and click through all links.
Make sure all pages look correct.
Turning in your lab
Your work will be evaluated on completeness and correction. Thoroughly test your code before you
turn it in. It is your responsibility to ensure correct files are turned in. You will NOT receive any
credit if wrong files are turned in even if you have completed the lab.
1. Zip the entire Lab01 folder and save it as lab01.zip.
2. Upload the lab01.zip file in Canvas.
Grading rubric
Your TA/instructor will assess your lab according to the following grading rubric. You should very
closely follow the instructions in this handout when working on the lab. Small deviations may be
fine, but you should avoid large deviations. You will not receive credits if your deviation does not
satisfy an item of the grading rubric. Whether a deviation is small or large and whether it satisfies
the requirement are at your TAs’ discretion. Here is the breakdown of the scoring:
Modifying the index.html file (5 points)
Activities Points
Create the hyperlinks in the navigation bar 0.5
Insert the logo image into the first cell in the banner 1
Create the h1 and h3 headings for the banner text 1
Create the h2 heading and paragraph inside the main body 1
Create the unordered list 1
Create the horizontal rule and paragraph in the footer 0.5
Creating the books.html file (5 points)
I210 Information Infrastructure I
Copyright © Louie Zhu 7
Activities Points
Change the h2 heading to “Books in Our Store” 0.5
Create the table with correct settings 3
Fill the table with content 1
Create hyperlinks in the table 0.5
Creating the civilwarbattlefieldguide.html file (5 points)
Activities Points
Change the h2 heading to “The Civil War Battlefield Guide” 0.5
Create the table with correct settings 2
Merge seven cells in the first column 1
Insert the image in the spanning cell 0.5
Fill the second and third column with correct content 1
Creating the greaterjourney.html file (5 points)
Activities Points
Change the h2 heading to “The Greater Journey: Americans in Paris” 0.5
Create the table with correct settings 2
Merge seven cells in the first column 1
Insert the image in the spanning cell 0.5
Fill the second and third column with correct content 1
Collepals.com Plagiarism Free Papers
Are you looking for custom essay writing service or even dissertation writing services? Just request for our write my paper service, and we'll match you with the best essay writer in your subject! With an exceptional team of professional academic experts in a wide range of subjects, we can guarantee you an unrivaled quality of custom-written papers.
Get ZERO PLAGIARISM, HUMAN WRITTEN ESSAYS
Why Hire Collepals.com writers to do your paper?
Quality- We are experienced and have access to ample research materials.
We write plagiarism Free Content
Confidential- We never share or sell your personal information to third parties.
Support-Chat with us today! We are always waiting to answer all your questions.
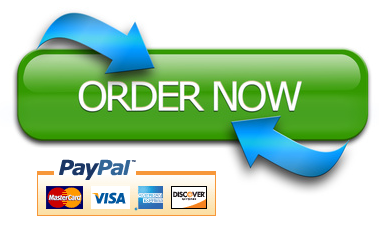