Per our last class… here are the changes/recommendations…
Per our last class… here are the changes/recommendations…
Building on the work you have already done on Assignment 2….
Basically this is the SAME THING as assignment 2 EXCEPT I will grade it more specifically and harshly and it needs to have significant Javascript edits in it.
Some of you already used javascript for the edits rather than HTML element attributes, so hopefully this will not be much work for you. For others, this may SEEM like a lot of work but keep in mind that MANY of the text fields will have the same sort of editing requirements, so you can reuse code or copy and paste it into new functions for each field and just statically name a function for each element you want to test, i.e. function firtname_check(), function mi_check(), function lastname_check()…
The difference is, you need to employ Javascript to validate and Warn the user if they do anything wrong in any of the fields AS THEY ENTER or LEAVE the field and AGAIN before they press the SUBMIT button. All the requirements of Homework 1 and 2 in terms of validation need to now be done with Javascript.
You can leave in the HTML checks, like MINLENGTH and PATTERNS, but you need to write a javascript version of a check for those conditions. This is a MUCH better technique for warning the user when they make mistakes (or when they are not giving you what you want).
DO NOT wait to do validation until a submit button is pressed.
DO NOT REMOVE but do not rely on the HTML validity edits. It may have value later, for homework 4. Belts and suspenders…
Leave the warnings/error messages on the page while there are errors and REMOVE the error messages IF the user corrects the info in that field.
Also, if you weren’t already doing this, make sure they have plenty of prompting as to your requirements for that element, using attributes like prompt and title.
Just in case you are still making local files:
The content must be hosted somewhere, either on an Oracle acount you set up OR an AWS or some other online account such as W3Spaces. You must give me the URL to go grade and NOT just zip up and upload the files to Canvas.
IF you were never able to get a w3spaces account or set up a public site that I could access, you need to let me know so I can try to get you a public account so you can FTP your files there. I host several public sites.
The html file should be named homework3.html ( do not obliterate your old homework files and do not put your student ID in the file name anymore.)
Remember all the requirements of homework 1 and 2:
MANDATORY: Make an EXTERNAL css file to refer to in your html document.
MANDATORY: Make an external javascript file(s) and reference your scripts in the original HTML page
Required Text boxes:
First Name (1 to 30 characters. ADVANCED EDITING: Letters, apostrophes and dashes only)
Middle Initial ( optional, 1 character, no numbers, blank/null ok)
Last Name (1 to 30 characters, ADVANCED EDITING: Letters, apostrophes and dashes only)
Date of Birth (3 pieces, MM/DD/YYYY) ADVANCED EDITING: must validate the date range, and nothing in the ftuture or more than 120 years ago)
Social Security (Do not use your real social when testing, must be 9 digits,ADVANCED EDITING: peformat as you type, i.e. stick in the dashes once 3 characters are typed). Alternatively, this can be an “id” number or come up with some reason to be asking for a 9 digit number, which should be obscured like the password field, but the field needs to be validated to have only numbers in it.
Address Line 1 (required,2 to 30 characters)
Address Line 2 (not required, but if entered, 2 to 30 characters)
City (required, 2 to 30 characters)
State (Give them a dropdown box with all 50 states, DC and PR in it including a NULL entry and NO default. Insert an Options list Snippet you find on the Internet)
Zip Code… .required, 5 digits only
Email address (ADVANCED javascript EDITING: must be in the format [email protected])
A text area to describe something.
Use the <TABLE> or STYLE structure to space things out nicely on the sceen – DO NOT WASTE SPACE ON THE SCREEN.
You need to include the following
Check Boxes
e.g. Check all of the following that apply: Have you had Chicken Pox, Measles, Covid-19, etc… at least 5
Radio Buttons, at least 3
e.g. own or rent, unsure
e.g. Have you been vaccinated.
Slide bar, at least 1 (ADVANCED EDITING: incrementing or decrementing a value that displays as you slide it.)
e.g. make a bar that asks for desired salary from $20,000/year to $200,00/year.
For real estate, make it as if the person wants to enter a rauge of prices to pay for a home.
You need to add a text box for a desired user ID and then 2 boxes for a passsword and a re-enter password.
You will need to create Javascript to VALIDATE the user name and password with these criteria:
ADVANCED EDITING: 1. user ID Can’t start with a number
2. ID Must be at least 5 characters but no more than 20
ADVANCED EDITING: 3. ID cannot have spaces or any special characters in it, just letters, numbers, the dash and the underscore ok.
4. Password fields must be secret/hidden on the form, i.e. typing produces **** instead of the characters.
5. Passwords must be at least 8 characters long.
ADVANCED EDITING: 6. Passwords must contain at least 1 upper case, 1 lower case letter and 1 digit.
7. Password CANNOT equal your desired User ID
8. Use 2 Password fields as shown in the class example. They must, of course, equal each other.
Feel free to ADD MORE if you choose. You are just trying to convince me that you know how to use and validate the most common form elements that a user encounters in a form.
Deciding WHEN to do the validation is up to you depending on the field type, i.e. a name or a password, and long as it is done prior to having to click a button. In other words, validate on the fly with, oninput, onfocus, onblur, etc…
If the user does anything wrong, you need to display a warning next to or underneath each item that they messed up on. It would be PREFERABLE not to cause the form to “jump around” when you add or remove warnings and instructions, so you should predefine some space on your page for these messages.
Be sure to set some error flags or keep an error count (or disable the submit button) so the user can’t submit bad data.
You can leave the “get data” button there if you made one in Homework 2 to redisplay all the data form without any editing. IF YOU WANT, you can
edit THAT routine to then ALSO call the javascript element validation functions and add that as another column in the output table… this would be just for debug puposes. You wouldn’t really do that in a real environment.
So… turning homework 2 into homework 3 (remember, do not overwrite old homework files)
REPLACE the traditional Submit button with a VALIDATE button which will call javascript functions to do the following:
Retrieve all the data from the form into the function (no need to redisplay it in a table unless you want to.)
Validate EVERY field, whether it is required or not (if not required and blank, you can skip it but if the user entered data in a field, it still has to be checked to make sure its not wierd characters.. use your best judgement as to what you think is weird or allowed depending on the field, i.e. names shouldn’t have anything but letters, spaces, apostrophes, etc…). Force lower case in fields like email address.
ONLY give the user an actual Submit button IF there are NO errors in any of the entered data on the form. Check this slick example on how to disable the submit button:
https://stackoverflow.com/questions/13931688/disable-enable-submit-button-until-all-forms-have-been-filledLinks to an external site.
The submit button will load a NEW html page that says: Thank you for your submission. We will be contacting you shortly.
YOUR DELIVERABLES in Canvas WILL BE
part 1:
Tell me the SPECIFIC URL to go to to see your work, i.e. profjake.w3spaces.com/MIS3371/homework3.html
Tell me the theme you chose.
part 2:
Give me a synopsis of what your website/form is supposed to be doing.
Describe to me what you are particularly happy with that you got working.
Also tell me what you DIDN’T YET get working.
If you prefer, you can upload an RTF file of part 2, so you can be more detailed and even include screenshots…. At least 100 words but not a ‘BOOK”.
part 3:
Attach and text document or PDF of the pseudocode you came up with, at least for the VALIDATE button so I can see your flow of logic to determine whether or not all the fields were good.
You can go look at my examples at profjake.w3spaces.com but just keep in mind, some of that code is intentionally broken.
You will be graded on BOTH the quality and detail of your answers as well as the actual presentation and funcationality of the page.
Collepals.com Plagiarism Free Papers
Are you looking for custom essay writing service or even dissertation writing services? Just request for our write my paper service, and we'll match you with the best essay writer in your subject! With an exceptional team of professional academic experts in a wide range of subjects, we can guarantee you an unrivaled quality of custom-written papers.
Get ZERO PLAGIARISM, HUMAN WRITTEN ESSAYS
Why Hire Collepals.com writers to do your paper?
Quality- We are experienced and have access to ample research materials.
We write plagiarism Free Content
Confidential- We never share or sell your personal information to third parties.
Support-Chat with us today! We are always waiting to answer all your questions.
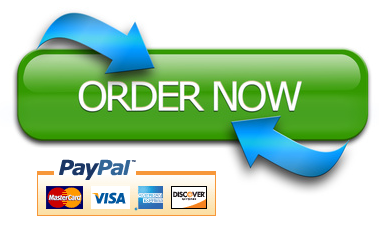