Closed Loop Control Lab
Closed Loop Control Lab Due: Tuesday, April 25, 2023 11:59pm CSCE317: Systems Engineering Introduction The objective of this lab is to write the necessary NIOS2 software and build the necessary hardware to implement a closed-loop controller to control the rotational speed of a DC motor that includes an integrated encoder. An encoder is a sensor that measures the rotational position or rotational speed of a spinning object; in this case, the internal motor shaft. Your software should accept the following parameters, defined in the code: 1. time: time to run the controller 2. T_s: controller time step 3. k_p, k_d, k_i: PID constants 4. setpoint: the speed desired for the external motor shaft in RPM The software can be written in a standard NIOS2 software project. You will need to add a parallel IO module in your platform design and connect it to the GPIO connector on the DE2-115 board. Outside the board, you will need to wire up an H-bridge and connect it to the motor. Additionally you will need to use the bench power supply to power the H-bridge and motor. The Motor The motor is a 6V DC motor equipped with a 50:1 gear box and a built-in Hall effect encoder that produces 12 pulses per revolution of its internal shaft. This translates to 600 pulses per revolution of the external shaft, due to the 50:1 gear box. The encoder provides two outputs are 180 degrees out of phase from one another as a means to determine the motor rotation direction. Figure 1: picture of motor It has six pins: 1. M1 (white wire): motor input 1, positive voltage relative to M2 drives the motor forward, negative voltage drives the motor backward 2. GND (purple wire) 3. C1 (green wire): first encoder input, pulses 12 time per revolution (will be high when C2 is low for forward revolution) 4. C2 (yellow wire): second encoder input, pulses 12 times per revolution (will be high when C1 is low for backward Copyright 2023 Jason Bakos, Philip Conrad 1 Closed Loop Control Lab Due: Tuesday, April 25, 2023 11:59pm CSCE317: Systems Engineering revolution) 5. VCC (black wire): connect to 6V 6. M2 (red wire): motor input 2, positive voltage relative to M1 drives the motor forward, negative voltage drives the motor backward The H-Bridge The H-bridge accepts two PWM signals on pins IA and IB, which each control the voltage delivered to its OA and OB pins. OA connects to motor’s M1 pin, OB connect to the motor’s M2 pin. Figure 2: picture of hbridge The H-bridge schematic is shown below. The IA pin controls switches S1 and S4, while the IB pin controls switches S2 and S3. The left and right side of the M block connect to the M1 and M2 pins of the motor. This way, when switches S1 and S4 are on, the M1 and M2 pins on the motor will receive a positive voltage. When switches S2 and S3 are on, the M1 and M2 pins will receive a negative voltage. Copyright 2023 Jason Bakos, Philip Conrad 2 Closed Loop Control Lab Due: Tuesday, April 25, 2023 11:59pm CSCE317: Systems Engineering Figure 3: hbridge schematic A PWM signal on IA will control the forward speed of the motor during which time the IB pin should be low. Likewise, a PWM signal on IB will control the backward speed of the motor during which time the IA pinshould be low.. The Encoder The motor’s encoder will produce a square wave, whose period (rising to rising edge or falling to falling edge) will give its rotational speed. The speed in revolutions per minute (RPM) is computed as: 1 (1 / period) / GEARING / PULSES_PER_ROTATION * 60, where GEARING = 50 and PULSES_PER_ROTATION = 12. Re-calculate the period on every rising edge of either C1 or C2. The direction of rotation can be determined by comparing the values of C1 and C2 whenever there is a rising edge on either. If C1 is high while C2 is low then then motor is moving forward, otherwise it is rotating backward. Copyright 2023 Jason Bakos, Philip Conrad 3 Closed Loop Control Lab Due: Tuesday, April 25, 2023 11:59pm CSCE317: Systems Engineering Pulse Width Modulation Your software can use either software-controlled PWM or hardware-controlled PWM. The example implementation below assumes a software-controlled PWM. You can use a hardcoded PWM period of 2048 clock cycles, which is approximately 24 KHz. The GPIO Connector on the DE2-115 Figure 4: GPIO connector Steps For Setting Up Your Project in Quartus, Platform I/O, and your software: 1. Add “inout logic [35:0] GPIO” to your list of top-level module I/Os 2. Add a parallel I/O to your platform design for a 2-bit output, called pwm_out 3. Add a parallel I/O input to your platform design for a 2-bit input, called encoder_in Copyright 2023 Jason Bakos, Philip Conrad 4 Closed Loop Control Lab Due: Tuesday, April 25, 2023 11:59pm CSCE317: Systems Engineering 4. In your top-level module, connect pwm_out to GPIO[1:0] 5. In your top-level module, connect encoder_in to GPIO[3:2] Steps For Setting Up Your Project on the Bench: 1. Place the H-bridge on the breadboard 2. From the power supply, connect channel 1’s positive terminal to the power supply positive row on the breadboard 3. From the power supply, connect channel 1’s ground terminal to the ground row on the breadboard 4. From the DE2-115’s expansion header, connect the pin on row 6 column 2 to the ground row on the breadboard 5. On the motor, connect VCC to the power supply positive row on the breadboard 6. On the H-bridge, connect pins 2 and 3 (middle pins, bottom row) to the power supply positive row on the breadboard 7. On the motor, connect GND to the ground row on the breadboard 8. On the H-bridge, connect pins 5 and 8 (outer pins, top row) to the ground row on the breadboard 9. From the DE2-115’s expansion header, connect pins 0 and 1 to pins 6 and 7 (middle pins, top row) on the H-bridge 10. From the DE2-115’s expansion header, connect pins 2 and 3 to the C1 and C2 on the motor 11. From the H-bridge, connect pins 1 and 4 (outer pins, bottom row) to the M1 and M2 pins on the motor Copyright 2023 Jason Bakos, Philip Conrad 5 Closed Loop Control Lab Due: Tuesday, April 25, 2023 11:59pm CSCE317: Systems Engineering Figure 5: Overall schematic Using the Power Supply Each bench station is equipped with a Keithley 2230G-30 195-Watt DC power supply with 3 channels that can supply up to 6 Amps or 60 Volts per output. For this project, we need a 6 V supply, with the motor requiring no more than 100 mA of current. To set up the power supply, follow these steps: 1. Turn the power supply on by pushing the green power button; note that this does not energize the output channels, as they will by OFF initially, 2. press the “CH1” button (assuming you want to use channel 1, although you can alternatively use channel 2), 3. press the V-Set button, 4. type “6.00” and press the “Enter” button, which is located in the center of the directional keys, 5. after approximately 3 seconds, the display will show “0.00 V” for the channel you just set, meaning Copyright 2023 Jason Bakos, Philip Conrad 6 Closed Loop Control Lab Due: Tuesday, April 25, 2023 11:59pm CSCE317: Systems Engineering that the voltage seen on that channel is currently zero. This is because the outputs are not turned on and there is no external voltage connected to the output channels, 6. when you are ready to activate the output channel, press the “(Output) On/Off” button, and then 7. watch the channel 1 voltage output, which should now show 6 Volts. Requirements Your Nios2 software should both control the motor and monitor its rotational speed and direction. The software should log the value of time, motor input, and motor speed and direction at 1/T_s Hz sampling rate, and then dump this log to the terminal before the program ends. The motor input should be represented as a duty cycle from -1 to 1 where positive values represent a duty cycle on the H-bridge’s IA pin with the IB pin set to 0 and negative values represent a duty cycle on the H-bridge’s IB pin with IA pin set to 0. The motor speed should be in revolutions per minute (RPM), with positive values representing forward rotation and negative values representing backward rotation. You may use your own definition of “forward” and “backward”. The program should run for time seconds, during which time it should cycle through the entire input signal. The relationshop between the motor input and speed, given by the encoder, is shown below: Copyright 2023 Jason Bakos, Philip Conrad 7 Closed Loop Control Lab Due: Tuesday, April 25, 2023 11:59pm CSCE317: Systems Engineering Figure 6: Overall schematic To implement your controller, you will need to choose values of k_p, k_d, and k_i to force the motor speed to the setpoint. Note that values of 0 are valid for any of these parameters. Controller Algorithm Your controller must be a PID controller and should perform control at a period defined by T_s. The error derivative and integral calculations may also be performed at this same period. 1 while True: 2 // read cycle counter 3 counter = read cycle_counter 4 5 // modulo by 2048 to create a counter that cycles at 50 MHz/2048 = ~24 KHz 6 pwm_progress = counter MOD 2^12 7 Copyright 2023 Jason Bakos, Philip Conrad 8 Closed Loop Control Lab Due: Tuesday, April 25, 2023 11:59pm CSCE317: Systems Engineering 8 // compute how many counts correspond to PWM duty cycle 9 duty_cycles = 2^14 * abs(motor_input) 10 11 // set PWM output, use PWM1 for reverse, PWM0 for forward 12 if speed < 0: 13 PWM_out1 = pwm_progress < duty_cycle ? 1 : 0 14 else: 15 PWM_out0 = pwm_progress < duty_cycle ? 1 : 0 16 17 // read encoder 18 encoder0_val = read encoder0 19 encoder1_val = read encoder1 20 21 // detect rising edge of encoder input 22 if prev_encoder0_val == 0 and encoder0_val == 1 23 encoder0_period = (counter – encoder0_rising_time) / CPU_FREQ 24 encoder0_rising_time = counter 25 if encoder1_val == 1: 26 direction = 1 27 else: 28 direction = 0 29 30 // detect rising edge of encoder input 31 if prev_encoder1_val == 0 and encoder1_val == 1 32 encoder1_period = (counter – encoder0_rising_time) / CPU_FREQ 33 encoder1_rising_time = counter 34 if encoder0_val == 1: 35 direction = 1 36 else: 37 direction = 0 38 39 // compute external shaft speed 40 current_motor_rpm = 1.0 / pulse_period / 12.0 / 50.0 * 60.0; 41 if direction==1: 42 current_motor_rpm = -current_motor_rpm 43 44 // record old encoder inputs 45 prev_encoder0_val = encoder0_val 46 prev_encoder1_val = encoder1_val 47 48 // determine if we should perform control 49 cycles_since_last_control = counter – prev_contol_action_cycles 50 time_since_last_control = cycles_since_last_control / CPU_FREQ Copyright 2023 Jason Bakos, Philip Conrad 9 Closed Loop Control Lab 51 52 53 54 55 56 57 58 59 60 Due: Tuesday, April 25, 2023 11:59pm CSCE317: Systems Engineering time_since_start = counter / CPU_FREQ // perform control if time_since_last_control >= T_s: error_old = error error = current_motor_rpm – setpoint error_accum += error error_delta = error-error_old motor_input = k_p * error + k_d * error_delta + k_i * error_accum 61 62 63 64 65 66 67 68 69 log time_since_start log motor_input log current_motor_rpm prev_contol_action_cycles = counter if time_since_start > time: stop_flag = 1 Submitting Your Code When you are ready to turn in your code for grading, each group should submit an archive of their project directory to Dropbox. To do this, enter the following commands: 1 rm -Rf /db /incremental_db / output_files 2 tar cvf lab3.tar 3 gzip lab3.tar Rubric • Bench setup = 40 points • Software interface to the DC motor = 20 points • Controller design = 20 points • Correct operation = 10 points • Data logging = 10 points Copyright 2023 Jason Bakos, Philip Conrad 10 Closed Loop Control Lab Due: Tuesday, April 25, 2023 11:59pm CSCE317: Systems Engineering Total points possible: 100. Copyright 2023 Jason Bakos, Philip Conrad 11
Collepals.com Plagiarism Free Papers
Are you looking for custom essay writing service or even dissertation writing services? Just request for our write my paper service, and we'll match you with the best essay writer in your subject! With an exceptional team of professional academic experts in a wide range of subjects, we can guarantee you an unrivaled quality of custom-written papers.
Get ZERO PLAGIARISM, HUMAN WRITTEN ESSAYS
Why Hire Collepals.com writers to do your paper?
Quality- We are experienced and have access to ample research materials.
We write plagiarism Free Content
Confidential- We never share or sell your personal information to third parties.
Support-Chat with us today! We are always waiting to answer all your questions.
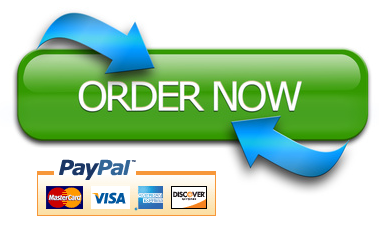