For this lab, you will need to make use of the following two dictionaries.
For this lab, you will need to make use of the following two dictionaries.
DAYS = {
0 : “SUN”,
1 : “MON”,
2 : “TUE”,
3 : “WED”,
4 : “THU”,
5 : “FRI”,
6 : “SAT”
}
HOURS = {
0 : “Mid-1AM “,
1 : “1AM-2AM “,
2 : “2AM-3AM “,
3 : “3AM-4AM “,
4 : “4AM-5AM “,
5 : “5AM-6AM “,
6 : “6AM-7AM “,
7 : “7AM-8AM “,
8 : “8AM-9AM “,
9 : “9AM-10AM “,
10 : “10AM-11AM”,
11 : “11AM-NOON”,
12 : “NOON-1PM “,
13 : “1PM-2PM “,
14 : “2PM-3PM “,
15 : “3PM-4PM “,
16 : “4PM-5PM “,
17 : “5PM-6PM “,
18 : “6PM-7PM “,
19 : “7PM-8PM “,
20 : “8PM-9PM “,
21 : “9PM-10PM “,
22 : “10PM-11PM”,
23 : “11PM-MID “,
}
This week we focus on our most significant bit of output to date and we will use the Pandas library to make our life easier.
Our goal is to implement print_temp_by_day_time() to print a table that shows the average temperature in our labs by day of week and hour of day. We use the get_avg_temperature_day_time() function that we just implemented.
The print_temp_by_day_time() function should first verify that the dataset object actually has a file loaded, using get_loaded_temps(). If not, it should complain and drop back to main().
You should build a dictionary. The keys of the the dictionary should be the days of the week (values of the above DAYS dictionary) and the values should be a list of average temperatures for each hour of the day.
Create a Pandas DataFrame from the dictionary you made. Make sure the index of the DataFrame is the hours of the day (keys of the HOURS dictionary) and the columns are the days from the DAYS dictionary.
A DataFrame is a 2-dimensional labeled data structure with columns of potentially different types. You can think of it like a spreadsheet or SQL table, or a dict of Series objects. It is generally the most commonly used pandas object.
Print out the header shown in the example, including the temperature units and the title, and the DataFrame.
The output should be very similar to the following table.
This specific table was printed with 4213 and 4218 active, be sure check and make sure you get the same results. Incidentally, you can see how this is useful – the temperature is getting pretty warm for study in the afternoons, and, and a bit chilly on Saturday evenings.
Average Temperatures for Test Week
Units are in Fahrenheit
SUN MON TUE WED THU FRI SAT
Mid-1AM 68.8 68.4 72.7 71.3 70.6 70.7 66.8
1AM-2AM 69.0 68.3 72.5 71.1 70.3 70.5 66.9
2AM-3AM 69.1 68.3 72.3 70.9 70.0 70.4 67.0
3AM-4AM 69.2 68.1 72.2 70.8 69.8 70.3 67.0
4AM-5AM 69.2 68.1 72.1 70.6 69.7 70.1 67.1
5AM-6AM 69.2 68.0 72.1 70.5 69.6 70.0 67.1
6AM-7AM 68.8 67.9 72.1 70.1 69.4 69.6 67.1
7AM-8AM 68.1 68.1 71.8 70.0 69.5 69.2 67.1
8AM-9AM 67.4 68.1 71.1 69.5 69.7 68.3 67.1
9AM-10AM 67.3 69.1 71.5 69.4 70.6 67.1 67.2
10AM-11AM 67.1 70.4 72.3 69.9 71.5 66.6 67.2
11AM-NOON 66.9 70.9 73.2 70.4 72.2 66.6 66.6
NOON-1PM 66.8 71.2 73.1 71.3 72.1 66.3 65.9
1PM-2PM 66.7 71.9 73.6 72.3 71.9 66.1 65.5
2PM-3PM 66.9 72.8 74.3 73.1 72.3 66.1 65.2
3PM-4PM 66.7 73.3 74.7 74.0 72.7 66.1 65.0
4PM-5PM 66.7 73.8 75.1 74.4 73.4 66.0 64.9
5PM-6PM 66.7 74.2 75.7 74.9 74.0 66.0 64.9
6PM-7PM 66.7 73.5 75.1 74.6 73.5 65.8 64.8
7PM-8PM 67.2 73.4 74.0 73.4 72.5 65.7 64.8
8PM-9PM 67.8 73.4 73.0 72.6 71.7 65.4 64.7
9PM-10PM 68.1 73.3 72.2 71.7 71.1 65.5 64.9
10PM-11PM 68.3 73.2 71.8 71.3 70.9 66.3 65.5
11PM-MID 68.6 73.0 71.5 70.9 70.8 66.6 65.7
Note that we included the name we gave the data file (in this case, Test Week). You should be using your name property to retrieve the name. Note that we identified the current units (using the dictionary, of course).
If data is not available at a particular day/time, the temperature should be replaced with “—” (not “None”). Unfortunately (or, I guess, fortunately) our data is complete, so the only way we can see this is if we print with all sensors turned off. But, your code should be checking one temperature at a time.
Also make sure the output has the proper precision setting and converts to the proper units.
Average Temperatures for Test Week
Units are in Fahrenheit
SUN MON TUE WED THU FRI SAT
Mid-1AM — — — — — — —
1AM-2AM — — — — — — —
2AM-3AM — — — — — — —
3AM-4AM — — — — — — —
4AM-5AM — — — — — — —
5AM-6AM — — — — — — —
6AM-7AM — — — — — — —
7AM-8AM — — — — — — —
8AM-9AM — — — — — — —
9AM-10AM — — — — — — —
10AM-11AM — — — — — — —
11AM-NOON — — — — — — —
NOON-1PM — — — — — — —
1PM-2PM — — — — — — —
2PM-3PM — — — — — — —
3PM-4PM — — — — — — —
4PM-5PM — — — — — — —
5PM-6PM — — — — — — —
6PM-7PM — — — — — — —
7PM-8PM — — — — — — —
8PM-9PM — — — — — — —
9PM-10PM — — — — — — —
10PM-11PM — — — — — — —
11PM-MID — — — — — — —
Make sure your code also converts the units properly
Collepals.com Plagiarism Free Papers
Are you looking for custom essay writing service or even dissertation writing services? Just request for our write my paper service, and we'll match you with the best essay writer in your subject! With an exceptional team of professional academic experts in a wide range of subjects, we can guarantee you an unrivaled quality of custom-written papers.
Get ZERO PLAGIARISM, HUMAN WRITTEN ESSAYS
Why Hire Collepals.com writers to do your paper?
Quality- We are experienced and have access to ample research materials.
We write plagiarism Free Content
Confidential- We never share or sell your personal information to third parties.
Support-Chat with us today! We are always waiting to answer all your questions.
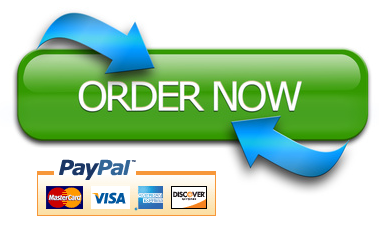