Python Question
We’re ready to implement another one of our menu items, and a couple of the instance methods of our class. We will be reading in a file that contains thousands of temperature readings taken during a week at the STEM Center.
DOWNLOAD THE FILE (STEM CENTER TEMPERATURE DATA DOWNLOAD STEM CENTER TEMPERATURE DATA) AND PLACE IT IN THE SAME DIRECTORY AS YOUR PYTHON FILES. THE FILE IS IN THE .CSV FORMAT (COMMA SEPARATED VALUE). EACH LINE REPRESENTS ONE “REPORT” FROM A SENSOR IN THE STEM CENTER, MOST OF WHICH ARE TEMPERATURE READINGS. IT IS A TEXT FILE, EACH FIELD REPRESENTS A VALUE AND THE FIELDS ARE SEPARATED BY A , (COMMA)
The format of each line is:
Day of Week, Time of Day, Sensor Number, Reading Type, Value
for example: 0 ,0.733263889, 0, TEMP, 17.59
Day of Week is an int where 0 represents Sunday, 1 represents Monday, etc.
Time of Day is a float between 0 and 1. 0 is 12:00AM and 1 is 11:59PM. The rest of the times are scaled linearly. We will convert this value to an hour of day value.
Sensor Number is an int, and maps to the sensors we coded in a previous assignment.
Reading Type is a string that contains “BATTERY”, “TEMP”, “AWAKE” or “SLEEPING”. We will only use the lines with “TEMP”.
Value is the value related of the reading. For TEMP type, this is degrees Celsius.
Steps to Implementation
This is a complex project and you will learn a lot. Here is a roadmap for what needs to be done:
First, create a new .py file for this assignment.
Copy in your code from Assignment 7, including it’s main().
Then copy the class TempDataset from Assignment 8. Make sure that you do not include the unit test from Assignment 8.
In main(), instantiate an object current_set of type TempDataset().
Update the function calls in our menu that pass the argument current_set. They currently pass the argument None, they should pass the dataset name.
Run this code to make sure you have no stray lines. Your menu should run just as in Assignment 7. Now we are ready to build on this code!
We will start with process_file(). This method has filename as one of its parameters. We need to try (hint) to open this file for reading. If we can’t open it, we need to return False. Once you have that coded, you must test it by calling process_file(“Temperatures2022-03-07.csv”) and process_file(“Blah”). Remember, these are instance methods in our class, so you need to call them using the current_set object we created. The first should quietly succeed if you have downloaded the data file and put it in the correct directory. The second should return False. If the unit-test code is retuned False,
Continuing in process_file(), recall that we have a variable _data_set in our class that was initialized to None. We want to reinitialize this as an empty list. Remember that this variable is an instance attribute in the class, be careful not to create another variable that is local to the method process_file()!
In a loop, we read-in each line from the file. We’ll need to do type conversions to make day and sensor as ints and temp as a float. We also must convert Time of Day to a number that represents the hour of the day. Multiply the given time by 24, and then use math.floor to round the result down to an integer between 0 and 23 (you will need to import the math library (import math) at the top of your module to use the floor function).
- We want to discard anything other than a temp reading (how?). For temperature readings, we will make a tuple:
- (day, time, sensor, temp)
and add the tuple to the list _data_set.
- We will assume that the data in the file is correct. We should be handling errors in the reading of the data, but for our purposes, if we get past opening the file, we’ll assume that everything else will go smoothly. Feel free to improve on this by returning False if any kind of load error happens throughout the process.
Continue until we are done with the list, and return True!
- Next we implement get_loaded_temps()
This method is simple. If we have not successfully loaded a data file, it should return None. Otherwise, it should return the number of samples that were loaded (an int). How we can check to verify that data file has been loaded, by looking at _data_set?
Finally, new_file()
- Recall that menu item 1 calls new_file(). This function should ask for a filename and then use process_file() to load the data.
If process_file() fails to load data, the program should complain (from new_file(), not from process_file!) to the user that it is unable to load a file. new_file() should then fall back to main.
- If process_file() succeeds, then new_file() should report the number of samples that were loaded.
NEW_FILE() SHOULD THEN ASK FOR A 3 TO 20 CHARACTER NAME FOR THE DATASET. USE THE SETTER METHOD WE CREATED IN TEMPDATASET TO VALIDATE AND SET THE NAME. TELL THE USER IF THE NAME IS BAD, AND DON’T LET THEM LEAVE UNTIL THEY INPUT A GOOD NAME. YOU ARE NOT CHANGING THE PROPERTY NAME() AT ALL, WE ALREADY VERIFIED THAT IT WORKS. ALL OF THIS FUNCTIONALITY SHOULD HAPPEN IN NEW_FILE().
Remember to change the menu routine, replace None in the call to new_file() with the appropriate object.
Note that process_file() and the property name() should not print anything.
Test the functionality of your loop, the number of samples retrieved should be the same as my sample run below (11,724).
One last thing. For testing, add these lines in main right after your call to print_menu():
if current_set._data_set is not None:
print([current_set._data_set[k] for k in range(0, 5000, 1000)])
After you run and load the file, you should see a list that looks (exactly) like this. The loop pulls out item 0, 1000, 2000, 3000 and 4000, and you can use this to verify that the data is loaded correctly. I will be adding this line to your code and checking that you have the correct data.
[(0, 18, 0, 17.5), (0, 2, 3, 21.59), (1, 9, 0, 19.55), (1, 12, 2, 23.23), (2, 4, 1, 22.05)]
We’re breaking the rules by directly accessing object data. So quickly take these lines out after you verify the data! We wouldn’t want to get caught.
That’s it! Make sure your sample run shows a failed load as well as a successful one.
Sample run:
STEM Center Temperature Project
Mike Murphy
Main Menu
———
1 – Process a new data file
2 – Choose units
3 – Edit room filter
4 – Show summary statistics
5 – Show temperature by date and time
6 – Show histogram of temperatures
7 – Quit
What is your choice? 1
Please enter the filename of the new dataset: Temperatures2022-03-07.csv
Loaded 11724 samples
Please provide a 3 to 20 character name for the dataset:
Main Menu
———
1 – Process a new data file
2 – Choose units
3 – Edit room filter
4 – Show summary statistics
5 – Show temperature by date and time
6 – Show histogram of temperatures
7 – Quit
What is your choice?
Collepals.com Plagiarism Free Papers
Are you looking for custom essay writing service or even dissertation writing services? Just request for our write my paper service, and we'll match you with the best essay writer in your subject! With an exceptional team of professional academic experts in a wide range of subjects, we can guarantee you an unrivaled quality of custom-written papers.
Get ZERO PLAGIARISM, HUMAN WRITTEN ESSAYS
Why Hire Collepals.com writers to do your paper?
Quality- We are experienced and have access to ample research materials.
We write plagiarism Free Content
Confidential- We never share or sell your personal information to third parties.
Support-Chat with us today! We are always waiting to answer all your questions.
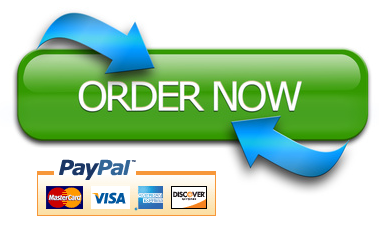