Python Question
In this Lab Assignment you will apply what we are learning about container objects (lists, tuples, sets, and dictionaries) to create and populate some container objects for our project. “Populate” in computer science means embedding objects into other objects.
We need a container object to store information about the sensors that are included in the STEM Center dataset. We will use this dataset in a later assignment. A dataset is a collection of data that is stored in a Python File Object, dataset it is a general descriptive term, not specific to Python. The Python file object represents a file stored on a hard disk drive or an SSD (solid state drive)
Our sensors are thermometers which placed in various rooms in the building and outside the building.
We will use two different container objects to store data and a third container object to keep track of which sensors we want to include in our reports (for instance, we might not be interested in the “outside” sensor or we might want to see data for just one room).
What type of “container” objects should we use? We will spend a moment thinking about data structures and design.
We are going to use 4 differents type of container objects in this class: lists, tuples, sets, and dictionaries. Each container object type has different features and benefits.
The information about each sensor that we are managing is very simple:
EACH SENSOR OBJECT IS A “CONTAINER” WHICH CONTAINS 3 PIECES OF INFORMATION ABOUT A SPECIFIC SENSOR.
the Sensor Number
the Room Number where a sensor is located
the Room Description, the name of the room
- How are we going to use this information?
- We want to be able to sort the sensors three different ways: By room number, by room description, or by sensor number.
- We want to be able to access sensor information by Room Number,
How do we choose which types of container objects to use: a list, tuple, set, or dict (dictionary)?
There are three choices:
- Just use a list containg tuples for the sensor information. [(0, “4213”, “STEM Center”), (1, “4201”, “Foundations Lab”, (2, “4204”, “CS Lab”), …]
- A tuple consists of items seperated by commas, each tuple is an object
(0, “4213”, “STEM Center”)
Sensor number = 0
Room number = “4213”
- Room name = “STEM Center”
(1, “4201”, “Foundations Lab”
- Sensor number = 1
- Room number = “4201”
Room name = “Foundations Lab”
- (2, “4204”, “CS Lab”)
Sensor number = 2
- Room number = “4204”
- Room name = “CS Lab”
- Use a dictionary for the sensor information
A dict (Dictionary object) consists of a key and a value, the key, in this case, is the Sensor number and the value is a tuple containing the Room number and Room name)
- 0:(“4213”, “STEM Center”)
- 1:(“4201”, “Foundations Lab”)
- 2:(“4204”, “CS Lab”)
- Use a dictionary, plus a list that mirrors all the information in the dictionary and gets sorted on its own
dict (dictionary object type)
- 0:(“4213”, “STEM Center”)
- 1:(“4201”, “Foundations Lab”)
- 2:(“4204”, “CS Lab”)
- list (list object type) , a parallel list that “points to” which item in the dictionary comes first, second, etc
[(1, “4201”, “Foundations Lab”), (0, “4213”, “STEM Center”), (2, “4204”, “CS Lab”)]
- It may seem odd to have the same information in a dictionary and a list. But remember that the order of the items in a Python dict object is not guaranteed by Python so we can’t rely on the dictionary to maintain the order of the items. We will cover the idea of an “ordered” dict later in the course.
So, for sorting, the dictionary will not work. This eliminates option two. But later we will be required to use a dictionary because we want to be able to find values based on a key, a tuple by itself will not work, this eliminates option one.
- Since the amount of data we are managing here is very small, only three fields, using a dictionary for random access to the sensors by Room Number and also maintaining a list that just points to items in the dictionary does not use a lot of memory.
- So it is decided, we will use option 3. You will create a dictionary with Room Number as the key, and the Room Description and Sensor Number as the value. After you have created the dictionary, I want you to use a list comprehension to create the corresponding list.
- The Program Spec
- For this assignment, we first need to let our program know about the temperature sensors that exist in the STEM Center. The sensors are referred to as ‘0’, ‘1’, ‘2’, etc. in the dataset, and it would be a lot to ask most humans to remember what sensor each number refers to. Here’s a handy sensor table for you:
Sensor NumberRoom NumberRoom Description04213STEM Center14201Foundations Lab24204CS Lab34218Workshop Room44205Tiled Room5OutOutside
Your first task is to put this information into a dict (dictionary) called sensors.
- READ AND UNDERSTAND THIS CAREFULLY:
The key for this dictionary is a string containing the room number. The value is a tuple containing the room description as a string in the first position, and the sensor number as an int in the second position.
- The next step is to create a list called sensor_list. Each item in this list is a tuple with the Room Number as a string in the first position, the Room Description in the second, and the Sensor Number in the third. You will use the sensors dictionary to load this list, do not code all the sensor information again. You should use a list comprehension to create this list from the dictionary. When you are done with the program, search for the number 4213. If it shows up more than once in your program, you have not done this part correctly.
- Since we are getting so good at list comprehensions, let’s create one more list that we will need later. Our data set will contain data from many different temperature sensors, and we want to choose which sensors to include when we present the information. filter_list will just be a list of integers, and in the beginning, it should contain all the sensors. Again, you should use a list comprehension to populate filter_list from the dictionary sensors. Do not use filter_list = [0,1,2,3,4,5] or a for loop with range().
- This code should be at the beginning of main(), after a docstring describing the fact that you are building the data structures required for the program.
- def main():
“”” Builds data structures required for the program
and provides the unit test code
“””
sensors = …
sensor_list = …
filter_list = …
Unit Test Code follows
I wrote this test code to help you determine if your containers are set up correctly. “Pass” for every category doesn’t mean everything is perfect, but if you see “Fail” for any category then there is definitely work to be done. Copy this code and paste it into main() as part of your unit test code,
- print(“Testing sensors:”)
if sensors[“4213”][0] == “STEM Center” and sensors[“Out”][1] == 5:
print(“Pass”)
else:
print(“Fail”)print(“Testing sensor_list length:”)
if len(sensor_list) == 6:
print(“Pass”)
print(“Testing sensor_list content:”)
for item in sensor_list:
if item[1] != sensors[item[0]][0]:
print(“Fail”)
break
else:
print(“Pass”)print(“Testing filter_list length:”)
if len(filter_list) == 6:
print(“Pass”)
else:
print(“Fail”)print(“Testing filter_list content:”)
if sum(filter_list) == 15:
print(“Pass”)
else:
print(“Fail”)
Here’s a sample run. Your sample run should look the same:
Testing sensors:
Pass
Testing sensor_list length:
Pass
Testing sensor_list content:
Pass
Testing filter_list length:
Pass
Testing filter_list content:
Pass
STEM Center Temperature Project
Mike Murphy
Main Menu
———
1 – Process a new data file
2 – Choose units
3 – Edit room filter
4 – Show summary statistics
5 – Show temperature by date and time
6 – Show histogram of temperatures
7 – Quit
What is your choice? 7
Thank you for using the STEM Center Temperature Project
Collepals.com Plagiarism Free Papers
Are you looking for custom essay writing service or even dissertation writing services? Just request for our write my paper service, and we'll match you with the best essay writer in your subject! With an exceptional team of professional academic experts in a wide range of subjects, we can guarantee you an unrivaled quality of custom-written papers.
Get ZERO PLAGIARISM, HUMAN WRITTEN ESSAYS
Why Hire Collepals.com writers to do your paper?
Quality- We are experienced and have access to ample research materials.
We write plagiarism Free Content
Confidential- We never share or sell your personal information to third parties.
Support-Chat with us today! We are always waiting to answer all your questions.
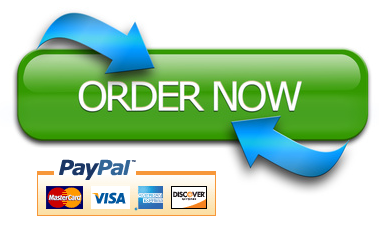