According to the Code Quality Guide, the program should have a class comment that describes what your program is doing at the beginning.
Please help me change the code according to the job requirements (pdf) and feedback (black bold font)
(DR) According to the Code Quality Guide, the program should have a class comment that describes what your program is doing at the beginning. See the Header and Class Comment section for what to include in these comments!
Reply
8 + import java.util.*;
9 +
10 + public class Budgeter {
11 + public static final int DAYS_IN_MONTH = 31;
12 +
13 + public static void main(String[] args) {
14 + Scanner console = new Scanner(System.in);
15 + intro();
(SD) Right now, main is not a concise summary of your program. Some of the contents in totalPart() should be in main instead. For example, getting the returns of the income total and expense totals is something that would make more sense for main to have, since its an important part of the program.
Reply
16 + totalPart(console);
17 +
18 + }
19 +
20 + // prints out the introduction of the program
21 + public static void intro (){
22 + System.out.println(“This program asks for your monthly income and”);
23 + System.out.println(“expenses, then tells you your net monthly income.”);
24 + System.out.println();
25 + }
26 +
(DR) Remember to comment on the return of any non-void method! This lets the reader understand what information they will receive from the method. See the Method Comments section of the Code Quality Guide for a couple examples of how to do this!
Reply
27 + // Reads in and computes the person’s income.
28 + //
29 + // Scanner console – the Scanner to use for input
30 + public static double totalIncome(Scanner console){
(SD) Notice how the code to compute the income/expense totals is almost exactly the same in your totalIncome() and totalExpense() methods! You could eliminate this redundancy by putting this code in a method with a String parameter for the “income” vs. “expense” cases, and calling it twice. See the Election program from lecture for an example of how to do this (make sure you are looking at the ElectionGood.java tab)!
Reply
31 + System.out.print(“How many categories of income? “);
32 + int numCate = console.nextInt();
33 + double totalInc = 0.00;
34 + for (int i = 1; i <= numCate; i++) {
35 + System.out.print(” Next income amount? $”);
36 + double incAmount = console.nextDouble();
37 + totalInc += incAmount;
(DR) We use indentation to show the reader the structure of our code. The indentation of a program should increase by one tab every time a curly brace “{“ is opened and decrease by one tab every time a curly brace “}” is closed. The Indenter Tool can help with this!
Reply
38 + }
39 + System.out.println();
40 + return totalInc;
41 + }
42 +
43 + // Reads in and computes the person’s expense.
44 + //
45 + // Scanner console – the Scanner to use for input
46 + public static double totalExpense (Scanner console){
47 + System.out.print(“Enter 1) monthly or 2) daily expenses? “);
48 + int numType = console.nextInt();
49 + double sum = 0.00;
50 + double totalExp = 0.00;
51 + System.out.print(“How many categories of expense? “);
52 + int numCate = console.nextInt();
53 + for (int i = 1; i <= numCate; i++) {
54 + System.out.print(” Next expense amount? $”);
55 + double expAmount = console.nextDouble();
56 + sum += expAmount;
57 + }
58 + if(numType == 1){
59 + totalExp = sum;
60 + }else if(numType == 2){
61 + totalExp = sum * DAYS_IN_MONTH;
62 + }
63 + System.out.println();
64 + return totalExp;
65 + }
66 +
67 + // Reads in and computes the person’s total income and expenses
68 + //
69 + // Scanner console – the Scanner to use for input
70 + public static void totalPart(Scanner console){
71 + double incTotal = totalIncome(console);
72 + double expTotal = totalExpense(console);
73 + double dailyInc = incTotal / DAYS_IN_MONTH;
74 + double dailyExp = expTotal / DAYS_IN_MONTH;
75 + System.out.println(“Total income = $” + round2(incTotal) +
76 + ” ($” + round2(dailyInc) + “/day)”);
77 + System.out.println(“Total expenses = $” + round2(expTotal) +
78 + ” ($” + round2(dailyExp) + “/day)”);
79 + System.out.println();
80 + double difference = incTotal – expTotal;
81 + if(difference > 0.00){
S
Shananda Dokka
TA
3 days ago
(UOLF) Notice how lines 83 and 88 are both the same. This means that they should not be in this nested conditional and should be factored before it instead.
Reply
82 + if(difference <= 250.00){
83 + System.out.println(“You earned $” + round2(difference) +
84 + ” more than you spent this month.”);
85 + System.out.println(“You’re a saver.”);
86 + System.out.println(“Well planned!”);
87 + }else{
88 + System.out.println(“You earned $” + round2(difference) +
89 + ” more than you spent this month.”);
90 + System.out.println(“You’re a big saver.”);
91 + System.out.println(“Awesome! You will be rich!”);
92 + }
93 + }else{
94 + if(difference <= –250.00){
(UOLF) Notice how lines 95 and 100 are both the same. This means that they should not be in this nested conditional and should be factored before it instead.
Reply
95 + System.out.println(“You spent $” + Math.abs(round2(difference)) +
96 + ” more than you earned this month.”);
97 + System.out.println(“You’re a big spender.”);
98 + System.out.println(“You need to plan your expenses well.”);
99 + }else{
100 + System.out.println(“You spent $” + Math.abs(round2(difference)) +
101 + ” more than you earned this month.”);
102 + System.out.println(“You’re a spender.”);
103 + System.out.println(“You should cut back a little bit.”);
104 + }
105 + }
106 + }
107 +
108 + // Returns the given number rounded to two decimal places.
109 + //
110 + // double num – the number to round
111 + public static double round2(double num) {
112 + return Math.round(num * 100.0) / 100.0;
113 + }
114 + }
Collepals.com Plagiarism Free Papers
Are you looking for custom essay writing service or even dissertation writing services? Just request for our write my paper service, and we'll match you with the best essay writer in your subject! With an exceptional team of professional academic experts in a wide range of subjects, we can guarantee you an unrivaled quality of custom-written papers.
Get ZERO PLAGIARISM, HUMAN WRITTEN ESSAYS
Why Hire Collepals.com writers to do your paper?
Quality- We are experienced and have access to ample research materials.
We write plagiarism Free Content
Confidential- We never share or sell your personal information to third parties.
Support-Chat with us today! We are always waiting to answer all your questions.
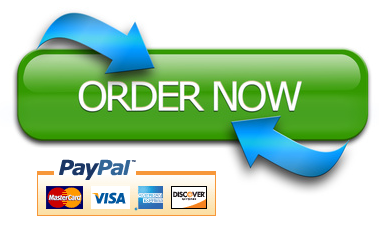