You have been hired at Marias Kitchen, a restaurant in Riverside. Chef and owner Maria has been asking her wait staff to take orders on paper.
You have been hired at Maria’s Kitchen, a restaurant in Riverside. Chef and owner Maria has been asking her wait staff to take orders on paper. She would like a new system where the wait staff can type in orders. The system should also print tickets for the kitchen staff that are easy to read so they know what dish to make and what table it belongs to.
For those of you who have food service experience, you will recognize this as a very simple point-of-sale (POS) and kitchen display system (KDS). Learn more about POS and KDS here.
Industry note: real systems tie into the restaurant’s financial and accounting records for auditing (finance and accounting majors), automatically reorder supplies and ingredients at the right time (supply chain majors), and allow access from a dashboard that can analyze the data for patterns and trends as well as employee performance (management, marketing, and business analytics majors).
Project 2: Maria’s Kitchen
The challenge in this Project is to use what you’ve learned in class and apply it to different case scenarios for both coding and the essay questions. In industry, there are no multiple choice questions where you can guess your way to an answer. You’ll have to generate real output (business model, financial or accounting statements, burndown charts, etc.) and present your argument as to why your team, company, or stakeholders should follow your output.
copy the starter code from there into your own repl. Don’t change any of the provided code.
def ticket(table, type, dish):
# Finish the rest of this function
def process(order):
# Finish the rest of this function
# Remember to add a blank line after the end of your process() function code. Start your "main" block of code below this line.
In the main part of your code outside of the functions
You need to create this code.
Ask the user to type in an order in this format (without the square brackets):
[table number]*[a, m, d, or f]-[the name of the dish]
The number is the table number. This is followed by a star.
Next comes a, m, d, or f, in lowercase. 'a' means appetizer. 'm' means main dish. 'd' means dessert. 'f' means 'on the fly' which tells the kitchen to cook that dish urgently.
This is followed by a dash.
At the end is the name of the dish.
For example:
2*m-burger and fries
This means table number 2, it is a main dish, and the dish is called 'burger and fries.'
The user will type all of this on one line with no spaces except for in the dish name.
Store this order into a list.
Let the user keep typing in orders. Store all orders in the same list. The user stops entering orders by typing in ‘x’ without quotes.
Once you have all of the orders, access each order in the list and send it to a function called process() that takes in the order as a parameter.
Send each order to a function called process(order)
You need to create the code for this function.
The function separates out the various components of the order: table number, type of order, and the dish name.
You need to send these three components to another function that will return a string.
Send these three components to a function called ticket(table, type, dish)
You need to create the code for this function.
The output of this function is a string with the order details that is nicely formatted so the kitchen staff can easily read it.
First, add “T“, the table number, and a space to the string.
Next, process the order type and dish name.
If it is an appetizer: add the word “APP”, a space, and the dish name to the string.
If it is a main dish: add the word “MAIN”, a space, and the dish name to the string.
If the length of the dish name is 10 characters or fewer, add the text “8 min” to the string to let the kitchen know how long to expect for the cook time.
If the length of the dish name is more than 10 characters, add the text “15 min” to the string.
If it is a dessert: add the word “DES”, a space, the dish name, and the word “BIRTHDAY” to the string to remind the pastry chef to add a special birthday message. Maybe today is Jing’s birthday? 🙂
If it is an “on the fly” order: add the word “OTF”, a space, and the dish name in all caps. For any “on the fly” orders, you don’t have to worry about the main dish or dessert string additions.
Using our example from above, the order “2*m-burger and fries” would result in a string:
T2 MAIN burger and fries 15 min
Return this string back to process().
Back inside process()
You need to continue creating the code for this function.
Store the string returned from ticket(). Print it for the user.
process() does not need to return anything.
Back inside the main part of your program
This kicks the program back down to the main part (outside of the functions) where your code should automatically pull the next order from the list and send it to process(). You need to create this functionality.
Once you’ve processed all the orders, your program can end and show a funny message to the wait staff (e.g., your TA).
Assumptions, clarifications, and hints
– Use the cheat sheet to learn how to separate a string into parts.
– The table number can be more than one digit. Therefore, don’t try to get the table number by indexing the first character of the order. Hint: cheat sheet.
– The order type (a, m, d, or f) will always be one lowercase letter; however, we don’t recommend indexing to get that either. Hint: cheat sheet. You want to allow for flexibility in case – Chef Maria adds more order types in the future that may have two or more characters.
– The dish name can contain spaces. You don’t have to worry about capitalization. The rest of the order will be typed in without spaces.
– You can assume that the wait staff (e.g., your TA) will accurately type in an order.
– Reminder: the user can type in as many orders as they want until they enter ‘x’. You have worked with this before in class.
Short answer questions: choose two
Choose two of the questions below to answer. Your answer for each question should be about half a page long, single-spaced. If you want to write more, the maximum length is one page, single-spaced. Include your references below your answer (references can also go on the next page). There are no specific guidelines for references, i.e., MLA vs. APA. You can just list the source where you found the information. Please use the default font size of Arial 11 and the default margins and spacing set by Google Docs.
Type your answers into the same Google Doc where you have your repl link. The link should be at the top of the page. Make sure the link is clickable and turns blue.
Pretend that you work in a hospital. It’s not really possible to have an MVP for something like surgery. For example, you can’t complete half a surgery, check for market feedback, then go back and do the other half of the surgery. Many surgeries and procedures need to be done in one shot. With this in mind, is it possible to use agile and MVP principles to make medical procedures more efficient? Is there anything you can do to help a large organization like a hospital go from bloated business (non-medical) processes to faster, more agile processes? Justify your answers with research.
Cloud computing is wonderful for the many benefits it provides. If you are reading this Doc, you are fortunate enough to have access to the Internet and a device. However, not everyone in the world has this opportunity. Pretend you work in the government of your home country. Identify some populations or areas that do not have access to cloud computing. Propose one or two solutions to bring cloud computing / Internet access to these populations or areas. Justify your answers with research.
Question 3 starts here and has multiple parts:
Do you think tech companies know information about you that you have not explicitly provided, such as gender (if you are comfortable sharing this in your answer), sexual orientation (if you are comfortable sharing this in your answer), what your job is, etc.?
If yes to the question above, provide two examples and identify your behaviors in technology usage that would let these companies find out (or assume) this information.
If no to the question above, provide one or two examples of how you are using technology while protecting your privacy.
Choose one of the positions below and defend your answer using opinions or research.
a. I believe it is a good thing that companies are collecting so much data on users because this helps the company build better and more useful products, like for health.
b. I think it is harmful for companies to be collecting so much data on users.
Question 3 ends here.
We understand that you learned in your writing classes that you should present your position and also acknowledge the opposing position. For question 3, please only present your position and make it clear for the reader what your position is.
Test case input and output
Your TA will create their own dish names and enter the following input:
Table 1, appetizer
Table 2, main dish with a length fewer than or equal to 10 characters
Table 3, main dish with a length greater than 10 characters
Table 456, dessert
Table 78, “on the fly”
Your TA can enter the information above in any order (position) they choose.
Here is my input and output. Your TA may choose different dish names and enter tables in a different order. This shouldn’t matter if your program is designed correctly. You don’t need the “!!! PRINTING KITCHEN TICKET !!!” line, I just added that to separate my output for readability.
HELLO WONDERFUL MARIA’S KITCHEN WAIT STAFF
Enter orders one at a time or x to quit
Order: 2*m-fried rice
Order: 1*a-cucumber salad
Order: 456*d-cheesecake
Order: 2*m-xiao long bao
Order: 78*f-side of ranch
Order: x
!!! PRINTING KITCHEN TICKET !!!
T2 MAIN fried rice 8 min
T1 APP cucumber salad
T456 DES cheesecake BIRTHDAY
T2 MAIN xiao long bao 15 min
T78 OTF SIDE OF RANCH
Hey TA, what is Peter Pan’s favorite restaurant? Wendy’s! 😀
Collepals.com Plagiarism Free Papers
Are you looking for custom essay writing service or even dissertation writing services? Just request for our write my paper service, and we'll match you with the best essay writer in your subject! With an exceptional team of professional academic experts in a wide range of subjects, we can guarantee you an unrivaled quality of custom-written papers.
Get ZERO PLAGIARISM, HUMAN WRITTEN ESSAYS
Why Hire Collepals.com writers to do your paper?
Quality- We are experienced and have access to ample research materials.
We write plagiarism Free Content
Confidential- We never share or sell your personal information to third parties.
Support-Chat with us today! We are always waiting to answer all your questions.
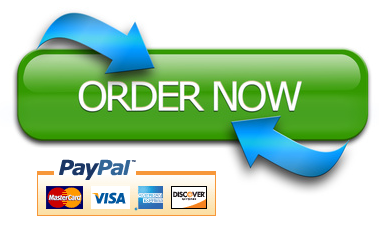