Model a real-life object as a Java class with at least one attribute and an instance method. Write a main method to create an instance of
1. Model a real-life object as a Java class with at least one attribute and an instance method. Write a main method to create an instance of the class, assign a value to the attribute, call your method, and demonstrate that the value of the attribute changed and that the method successfully completed what it was supposed to do. The main method can be created in a separate driver class (e.g. MyDriver.java) within which you will create an instance of the object class. For example, if the name of the object class is Car (meaning, you are coding Car.java) then you can create an instance of Car inside MyDriver.java.
Be sure to create a program different from any of the programs already posted by your classmates or the examples in-class materials.
2. Reply to another student's post. Modify the student’s class to add another attribute and a method that fits (is consistent with) the object the class is modeling, or suggest a different version of the code. Write the main method to demonstrate the correct functionality of the additions. As you reply to the other students, try to reply to a post that does not have a reply yet, and if not; try to reply to a post with a fewer number of replies.
Be sure to create a program different from any of the programs already posted by your classmates or the examples in the class materials.
As you answer these questions, use proper Java naming convention (Camel case), name the class, attribute, and method in a meaningful way to represent the business meaning, and add comments to the Java code as applicable.
The deliverables are the Java code and the documentation. The documentation is a single Microsoft Word document, or PDF containing the screenshot of the results obtained by running the code.
Assignment 1
Object and Instance
Before attempting this assignment, be sure you have completed all the reading assignments, non-
graded exercises, discussions, and assignments to date.
Overview This assignment consists of two classes that will be implemented. A Weight.java class that has
three private variables, two private methods and four public methods and a Project.java class
that has three private methods. The goal of this assignment is to perform calculations using
Weight objects (instances of Weight class).
Weight Class Weight class should have three private variables, two private methods and four public methods.
Variables
Hint: How should/can you access these private variables?
1. A private constant variable called OUNCES_IN_A_POUND that defines the number of ounces in a pound (16).
2. A private variable called pounds with a data type of integer.
3. A private variable called ounces with a data type of double.
Methods
1. A public parameterized constructor, which initializes the private variables.
public Weight (int pounds, double ounces){ // implementation
}
2. A private instance method called toOunces with a return type of double. This method has no parameters and should return the total number of ounces. For full credit, reuse this method
across other methods when possible.
3. A private instance method called normalize with a return type of void. This method has no parameters and should ensure that the number of ounces is less than the number of ounces in a
pound. For full credit, reuse this method across other methods when possible.
4. A public instance method called lessThan with a return type of boolean. This method should accept a Weight object as a parameter and determine if the object is greater or less than
the initialized values.
public boolean lessThan (Weight weight){ // implementation
}
5. A public instance method called addTo with a return type of void. This method should accept a Weight object as a parameter and add the object’s weight values to the initialized
values.
public void addTo (Weight weight){ // implementation
}
6. A public instance method called toString with a return type of String. This method has no parameters and should have the following format:
x pounds and y ounces
where x is the number of pounds and y the number of ounces. Ounces should be
displayed with two decimal places.
Project1 Class Project1 class should have three private methods and one public method.
Methods
1. A private class method named findMinimum with a return type of Weight. This method should accept three Weight objects as parameters and compare each Weight object’s
weight values to find the minimum. The Weight object with the minimum weight value
should be returned and then printed using toString in the following format:
The minimum weight is x pounds and y ounces
where x is the number of pounds and y the number of ounces. Ounces should be
displayed with two decimal places.
2. A private class method named findMaximum with a return type of Weight. This method should accept three Weight objects as parameters and compare each Weight object’s weight
values to find the maximum. The Weight object with the maximum weight value should be
returned and then printed using toString in the following format:
The maximum weight is x pounds and y ounces
where x is the number of pounds and y the number of ounces. Ounces should be
displayed with two decimal places.
3. A private class method named findAverage with a return type of Weight. This method should accept three Weight objects as parameters and calculate the average weight value. A new
Weight object with the average weight values should be returned and then printed using toString
in the following format:
The average weight is x pounds and y ounces
where x is the number of pounds and y the number of ounces. Ounces should be
displayed with two decimal places.
4. A public method named main with a return type of void. This method should exercise the correct functionality of findMinimum, findMaximum and findAverage by creating three
Weight objects using the hardcoded values below:
Weight weight1 = new Weight(11, 3); Weight weight2 = new Weight(7, 20); // Hint: normalize method should be used to translate into 8 pounds and 4 ounces Weight weight3 = new Weight(14, 6);
Submission Requirements
Style and Documentation Make sure your Java program is using the recommended style such as:
• Javadoc comment with your name as author, date, and brief purpose of the program
• Comments for variables and blocks of code to describe major functionality
• Meaningful variable names and prompts
• Class names are written in upper CamelCase
• Constants are written in All Capitals
• Use proper spacing and empty lines to make code human readable
Deliverables The submission requires uploading a single zip file, which will contain:
1. The source code of Weight.java (do not include the class file) 2. The source code of Project1.java (do not include the class file) 3. A single document (DOC/DOCX or PDF), which includes the test case(s) in a tabular
format and screenshot(s) of the test runs for the test case(s). Do not submit the
screenshot(s) separately as they should be included in this document. Below is an
example of a test case table to follow:
Test # Input Values
(Values as Input)
Expected Output Actual
Output
Pass or
Fail
(What is Expected as
Result)
(Output
obtained by
code
execution)
(If test
passed or
failed)
Test 1 Weight weight1 =
new Weight(11, 3)
Weight weight2 =
new Weight(7, 20)
Weight weight3 =
new Weight(14, 6)
Created weight1 with 11
pounds and 8 ounces
Created weight2 with 8
pounds and 4 ounces
Created weight3 with 14
pounds and 6 ounces
Screenshot(s)
of the Output
(Result
obtained by
executing the
code)
Include the screenshot(s) here
Grading Rubric Criteria Level 3 Level 2 Level 1
Weight
class
(31-40 points)
Implemented the
specifications and logic
components
(21-30 points)
Not implemented some of
the logic and specification
components
(0-20 points)
Not implemented most
of the logic and
specification
components
Project1
class
(31-40 points)
Implemented the
specifications and logic
components
(21-30 points)
Not implemented some of
the logic and specification
components
(0-20 points)
Not implemented most
of the logic and
specification
components
Test
cases
(11-20 points)
Included the test cases
with the screen shot(s)
using the tabular format
(6 – 10 points)
Not included some of the
test case using the screen
shot(s) using the tabular
format
(0 – 5 points)
Not included most of
the test cases with the
screen shot(s)
,
Assignment 2: Inheritance and Encapsulation
Before attempting this project, be sure you have completed all the reading assignments, non-graded
exercises, discussions, and assignments to date.
Design and implement Java program as follows:
(1) There will be a Snack class with following attributes: id (combination of numbers and letters),
size (values S, M, or L), and price
(2) There will be two child classes FruitSnack and SaltySnack with the following additional
attributes:
o FruitSnack: It includes citrus fruit indication (value of this variable of is true or false)
o SaltySnack: It includes nut snack indication (value of this variable of is true or false)
(3) The Snack class hierarchy must provide the following functionality:
• On creation, a snack instance must be given all attribute values except its price, which must be calculated
• Price is calculated as follows: o There is a flat fee of $19.99 for S snack, $29.99 for M snack, and $39.99 for L snack.
o FruitSnack has an additional fee of $5.99 when it has a citrus fruit. Please add
only a single citrus fruit, and no preventing coding is required to limit adding more
than one.
o SaltySnack has an additional fee of $4.50 when it has a nut snack. Please add
only a single nut snack no preventing coding is required to limit adding more than
one.
• Each class must have a method to return or display the class’s values to the console
(4) Implement OrderSystem class with main method with following functionality:
o Order a snack and after ordering it will display the snack type, its size, id and price
o Exit program
(5) Your classes must be coded with correct encapsulation: private/protected attributes, get methods,
and set methods
(6) There should be appropriate overloading and overriding methods
(7) OrderSystem should take advantage of the inheritance properties (e.g., use Snack variable
regardless which snack instance as appropriate)
(8) The prices for S, M, L, citrus fruit, nut snack can be hard coded in the program.
Style and Documentation:
Make sure your Java program is using the recommended style such as:
• Javadoc comment with your name as author, date, and brief purpose of the program
• Comments for variables and blocks of code to describe major functionality
• Meaningful variable names and prompts
• Class names are written in upper CamelCase
• Constants are written in All Capitals
• Use proper spacing and empty lines to make code human readable
Capture execution:
You must capture the screen shots of running the code for different test scenarios and include the
screen shots of the test runs in the documentation. The following is a sample of a few of the test
scenarios for you to follow, and you can add more. Any variation with the menu shown below, or any
change in the language of the display or the display format for the menu for a justified reason is
accepted.
In the test scenarios the symbol of question mark ( ?) means that is the value your program will
compute. For example, your program will display the value of id, that is noted as ?
Your program will compute the value of the price and display it, and that is also indicated as ?.
Test Scenario 1: MENU 1: Order a Snack 2: Exit program Enter your selection: 2 Thank you for using the program. Goodbye!
Test Scenario 2: MENU 1: Order a Snack 2: Exit program Enter your selection: 1 Do you want Fruit Snack (1) or Salty Snack (2): 1 What size do you want: S, M, or L: S Do you want citrus fruit included? true/false: true You have chosen snack type = Fruit Snack, of type = S, id = ? and price = ?
Test Scenario 3: MENU 1: Order a Snack 2: Exit program Enter your selection: 1 Do you want Fruit Snack (1) or Salty Snack (2): 1 What size do you want: S, M, or L: M Do you want citrus fruit included? true/false: false You have chosen snack type = Fruit Snack, of type = M, id = ? and price = ?
Test Scenario 4: MENU 1: Order a Snack 2: Exit program Enter your selection: 1 Do you want Fruit Snack (1) or Salty Snack (2): 1 What size do you want: S, M, or L: L Do you want citrus fruit included? true/false: false You have chosen snack type = Fruit Snack, of type = L, id = ? and price = ?
Test Scenario 5: MENU 1: Order a Snack 2: Exit program Enter your selection: 1 Do you want Fruit Snack (1) or Salty Snack (2): 2 What size do you want: S, M, or L: S Do you want nut snack included? true/false: true You have chosen snack type = Salty Snack, of type = S, id = ? and price = ?
Test Scenario 6: MENU 1: Order a Snack 2: Exit program Enter your selection: 1 Do you want Fruit Snack (1) or Salty Snack (2): 2 What size do you want: S, M, or L: M Do you want nut snack included? true/false: false You have chosen snack type = Salty Snack, of type = M, and id = ? and price = ?
Submission Requirements:
Deliverables include Java program (.java files) and a single documentation including the screen shots
of the test runs either as a Microsoft Word file, or as a PDF. The Java and Word/PDF files should be
named appropriately for the assignment (as indicated in the Submission Requirements document.
The word (or PDF) document should include screen captures showing the successful compiling and
running of each of the test scenario. Test scenarios should include all required functionality of the
program. Each screen capture should be properly labeled clearly indicated what the screen capture
represents. Do not submit the screen shots of the test runs separately as image files but include
(embed) them in the document and finally submit a single document.
Grading Rubric:
The following grading rubric will be used to determine your grade:
Criteria Level 3 Level 2 Level 1
The Snack hierarchy
classes (21-30 points)
Correct or almost
correct attributes and
inheritance structure
(11-20 points)
Mistakes in
implementation
(0-10 points)
Missing or
significantly incorrect
implementation
Encapsulation (21-30 points)
Correct or almost
correct code for
encapsulation
(11-20 points)
Mistakes in
implementation
(0-10 points)
Missing or
significantly incorrect
implementation
Calculation (8-10 points)
Correct or almost
correct code to
calculate price using
overriding
(6-7 points)
Mistakes in
implementation
(0-5 points)
Missing or
significantly incorrect
implementation
Test cases (11-15 points)
Correct or almost
correct code to meet
required functionality
(6-10 points)
Mistakes in
implementation
(0-5 points)
Missing or
significantly incorrect
implementation
Program
documentation and
style, screen captures
(11-15 points)
Correct or almost
correct menu,
program comments,
(6-10 points)
Mistakes or
incomplete menu,
documentation and/or
(0-5 points)
Missing or
significantly incorrect
menu, documentation
identifiers, and screen
captures
style, and screen
captures
and/or style, or screen
captures
,
Assignment 3
GUI & Polymorphism
Before attempting this project, be sure you have completed all the reading assignments, non- graded exercises, discussions, and assignments to date.
Design and implement Java program as follows:
1) Implement converter class hierarchy as follows: a. Converter class which includes:
▪ Private attribute for input of data type double ▪ Default constructor with no parameter which sets input to Double.NaN
▪ Overloaded constructor with input for parameter ▪ Get and set methods for input attribute ▪ Method convert() which returns input value
b. TemperatureConverter class which is a child of Converter and includes:
▪ Constructors which call parent constructors ▪ Overridden convert() method to convert input (Fahrenheit temperature) to
Celsius and returns the value. If the instance has no input value, it should return Double.NaN
▪ Use the following formula for conversion: C = ((F-32)*5)/9 c. DistanceConverter class which is a child of Converter and includes:
▪ Constructors which call parent constructors ▪ Overridden convert() method to convert input (distance in miles) to distance in
kilometers and returns the value. If the instance has no input value, it should return Double.NaN
d. Use the following formula for conversion: KM = M * 1.609 2) Implement GUIConverter class using JFrame and JPanel as follows:
a. GUI will have 3 buttons: “Distance Converter”, “Temperature Converter”, and “Exit”.
b. When user clicks Exit, the program will terminate
c. When user clicks Distance Converter, an input dialog will pop up where user can type
value and click OK:
d. Once user clicks OK, message dialog will pop up:
e. When user clicks on Temperature button, an input dialog will pop up to input value
and then when clicks OK, the message dialog with pop up with converted result:
f. SUGGESTIONS: ▪ For the input dialog you can use JOptionPane.showInputDialog ▪ The ActionListener for each Converter button should create the appropriate
Converter child instance, set the input, and call its convert() method ▪ For the pop up with converted value you can use
JOptionPane.showMessageDialog
Style and Documentation:
Make sure your Java program is using the recommended style such as: ▪ Javadoc comment up front with your name as author, date, and brief purpose of
the program
▪ Comments for variables and blocks of code to describe major functionality
▪ Meaningful variable names and prompts ▪ Class names are written in upper CamelCase
▪ Constants are written in All Capitals ▪ Use proper spacing and empty lines to make code human readable
Capture execution: You should capture and label screen captures associated with compiling your code, and running a passing and failing scenario for each functionality
Submission requirements
Deliverables include Java program (.java) and a single Word (or PDF) document. The Java files should be named appropriately, and the Word/PDF file should be named as Assignment3.
The word (or PDF) document should include screen captures showing the successful compiling and running of each of the test scenario. Test scenarios should include all required functionality
of the program. Each screen capture should be properly labeled clearly indicated what the
screen capture represents.
Submit your files to Assignment 3 submission area no later than the due date listed in your online classroom.
Grading Rubric:
The following grading rubric will be used to determine your grade:
Attribute Level Level Level 0
(15-20 points) (5-15 points) (0 – 5 points)
The Converter class Correct or Mistakes in Missing or significantly
hierarchy almost correct implementation incorrect implementation
attributes and
inheritance
structure and
polymorphism
(overloading and
overriding)
Welcome screen Correct or Mistakes in Missing or significantly
with buttons almost correct implementation incorrect implementation
code to display
three buttons
Distance Converter Correct or Mistakes in Missing or significantly
almost correct implementation incorrect implementation
code for distance
converter button
Temperature Correct or Mistakes in Missing or significantly
Converter almost correct implementation incorrect implementation
code for
temperature
converter button
Program Correct or Mistakes or Missing or significantly
documentation and almost correct incomplete menu, incorrect menu, documentation
style, screen menu, program documentation and/or style, or screen captures
captures comments, and/or style, and
identifiers, and screen captures
screen captures
,
Project
Media Rental System
Before attempting this project, be sure you have completed all of the reading assignments, non-
graded exercises, examples, discussions, and assignments to date.
Design and implement Java program as follows:
1) Media hierarchy:
Create Media, EBook, MovieDVD, and MusicCD classes from Week 3 -> Practice Exercise – Inheritance solution.
Add an attribute to Media class to store indication when media object is rented versus available. Add code to constructor and create get and set methods as appropriate.
Add any additional constructors and methods needed to support the below functionality
2) Design and implement Manager class which (Hint: check out Week 8 Reading and Writing files example):
stores a list of Media objects
has functionality to load Media objects from files
creates/updates Media files
has functionality to add new Media object to its Media list
has functionality to find all media objects for a specific title and returns that list
has functionality to rent Media based on id (updates rental status on media, updates file, returns rental fee)
3) Design and implement MediaRentalSystem which has the following functionality:
user interface which is either menu driven through console commands or GUI buttons or menus. Look at the bottom of this project file for sample look and feel. (Hint: for
command-driven menu check out Week 2: Practice Exercise – EncapsulationPlus and
for GUI check out Week 8: Files in GUI example)
selection to load Media files from a given directory (user supplies directory)
selection to find a media object for a specific title value (user supplies title and should display to user the media information once it finds it – should find all media with that
title)
selection to rent a media object based on its id value (user supplies id and should display rental fee value to the user)
selection to exit program 4) Program should throw and catch Java built-in and user-defined exceptions as appropriate 5) Your classes must be coded with correct encapsulation: private/protected attributes, get
methods, and set methods and value validation
6) There should be appropriate polymorphism: overloading, overriding methods, and dynamic binding
7) Program should take advantage of the inheritance properties as appropriate
Style and Documentation:
Make sure your Java program is using the recommended style such as:
Javadoc comment up front with your name as author, date, and brief purpose of the program
Comments for variables and blocks of code to describe major functionality
Meaningful variable names and prompts
Class names are written in upper CamelCase
Constants are written in All Capitals
Use proper spacing and empty lines to make code human readable
Capture execution:
You should capture and label screen captures associated with compiling your code, and running
the a passing and failing scenario for each functionality
Submission requirements
Deliverables include Java program (.java files) and a single Word (or PDF) document. The
Word/PDF files should be named appropriately for the assignment (as indicated in the
SubmissionRequirements document.
The word (or PDF) document should include screen captures showing the successful compiling
and running of each of the test scenario. Test scenarios should include all required functionality
of the program. Each screen capture should be properly labeled clearly indicated what the screen
capture represents.
Submit your files to project submission area no later than the due date listed in your online
classroom.
Grading Rubric: The following grading rubric will be used to determine your grade:
Attribute Level
(15-20 points)
Level
(5-15 points)
Level 0
(0 – 5 points)
Media hierarchy Correct or
almost correct
code to meet
project
requirements
Mistakes in
implementation
Missing or significantly
incorrect implementation
Manager Correct or
almost correct
code to meet
requirements
Mistakes in
implementation
Missing or significantly
incorrect implementation
MediaRentalSystem:
load data, find
media, rent media
Correct or
almost correct
code to meet
requirements
Mistakes in
implementation
Missing or significantly
incorrect implementation
Encapsulation,
polymorphism,
exception handling
Correct or
almost correct
code to meet
requirements
Mistakes in
implementation
Missing or significantly
incorrect implementation
Program
documentation and
style, screen
captures
Correct or
almost correct
menu/GUI,
program
comments,
identifiers, and
screen captures
Mistakes or
incomplete
menu/GUI,
documentation
and/or style, and
screen captures
Missing or significantly
incorrect menu/GUI,
documentation and/or style, or
screen captures
Sample User interface – command driven:
Welcome to Media Rental System 1: Load Media objects… 2: Find Media object… 3: Rent Media object… 9: Quit Enter your selection : 1 Enter path (directory) where to load from: blah File cannot be opened: Could not load, no such directory Welcome to Media Rental System 1: Load Media objects… 2: Find Media object… 3: Rent Media object… 9: Quit Enter your selection : 1 Enter path (directory) where to load from: C:/tmp-umuc Welcome to Media Rental System 1: Load Media objects… 2: Find Media object… 3: Rent Media object… 9: Quit Enter your selection : 2 Enter the title: blah There is no media with this title: blah Welcome to Media Rental System 1: Load Media objects… 2: Find Media object… 3: Rent Media object… 9: Quit
Enter your selection : 2 Enter the title: Forever Young EBook [ id=123, title=Forever Young, year=2018, chapters=20 available=true] MovieDVD [ id=126, title=Forever Young, year=2020, size=140.0MB available=false] Welcome to Media Rental System 1: Load Media objects… 2: Find Media object… 3: Rent Media object… 9: Quit Enter your selection : 3 Enter the id: 123 Media was successfully rented. Rental fee = $2.00 Welcome to Media Rental System 1: Load Media objects… 2: Find Media object… 3: Rent Media object… 9: Quit Enter your selection : 2 Enter the title: Forever Young EBook [ id=123, title=Forever Young, year=2018, chapters=20 available=false] MovieDVD [ id=126, title=Forever Young, year=2020, size=140.0MB available=false] Welcome to Media Rental System 1: Load Media objects… 2: Find Media object… 3: Rent Media object… 9: Quit Enter your selection : 3 Enter the id: 999 The media object id=999 is not found Welcome to Media Rental System 1: Load Media objects… 2: Find Media object… 3: Rent Media object… 9: Quit Enter your selection : 9 Thank you for using the program. Goodbye!
Sample User interface – GUI:
Above closed the window by pressing the X, results in below:
Collepals.com Plagiarism Free Papers
Are you looking for custom essay writing service or even dissertation writing services? Just request for our write my paper service, and we'll match you with the best essay writer in your subject! With an exceptional team of professional academic experts in a wide range of subjects, we can guarantee you an unrivaled quality of custom-written papers.
Why Hire Collepals.com writers to do your paper?
Quality- We are experienced and have access to ample research materials.
We write plagiarism Free Content
Confidential- We never share or sell your personal information to third parties.
Support-Chat with us today! We are always waiting to answer all your questions.
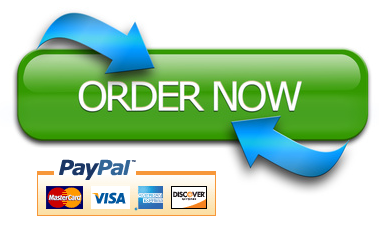