Read chapter 9.Modify the slot program from the book with the following changes:1. Add two extra windows in the stats group bo
Read chapter 9.Modify the slot program from the book with the following changes:1. Add two extra windows in the stats group box: one is called BET (this is the amount of dollars the user wants to bet on each game, amount cannot be more than the balance); the other is called WIN (this is the amount of money won by the user on each game, use a negative amount when it's a loss).2. Ask user how much money to start with instead of using Account =20 on the program. This will be the initial amount to place in Account window.3. – When user loses (i.e. 3 different pictures displayed), subtract 1x BET amount to the account. Display value in WIN with a negative amount. – When user wins 2 (i.e. 2 same pictures displayed), add 2x BET amount to the account. Display amount in WIN with a positive amount. - When user wins 3 (i.e. jackpot), add 5x BET amount to the Account. Display amount in WIN with a positive amount.Remember the Account value window calculates the user balance after each game.4. Add the following: User Wins and Game is over if: Account Window is 0 or les or Account Window is 10 times or more of user initial amount (For instance, let's say user starts playing with 30 and the Account window is 300 or more).Display a notice to show: how many games it takes the user to win and user Account balance.
* Remember to also submit a text file of the entire program (not an image), screenshots of the output, and the .bas file.
' ************************************************************************* ' ' Script Name: SlotMachine.bas (The Slot Machine Game) ' Version: 1.0 ' Author: Jerry Lee Ford, Jr. ' Date: March 1, 2007 ' ' Description: This game is a simulation of a Las Vegas-styled slot ' machine. The objective of the game is for the player to ' win as much money as possible and not go broke. ' ' ************************************************************************* nomainwin 'Suppress the display of the default text window 'Assign default values to global variables global iteration, account, gamesPlayed iteration = 0 'Used to control the display of animation account = 20 'Represents the amount of money in the player's account gamesPlayed = 0 'Keeps track of the total number of games played call ManageGamePlay 'Call the subroutine responsible for managing game play wait 'Pause the application and wait for the player's instruction 'This subroutine displays the game board and controls interaction with the 'player sub ManageGamePlay WindowWidth = 500 'Set the width of the window to 500 pixels WindowHeight = 500 'Set the height of the window to 500 pixels loadbmp "Cherry", "C:imagesCherry.bmp" 'Load the specified bitmap 'file into memory loadbmp "Apple", "C:imagesApple.bmp" 'Load the specified bitmap 'file into memory loadbmp "Pear", "C:imagesPear.bmp" 'Load the specified bitmap 'file into memory 'Define the format of statictext controls displayed on the window statictext #play.statictext1, "S L O T M A C H I N E", 77, 20, 440, 30 statictext #play.statictext2, "Copyright 2007", 345, 55, 80, 20 'Add the controls used to graphically display slot machine values graphicbox #play.pic1, 50, 100, 93, 93 graphicbox #play.pic2, 200, 100, 93, 93 graphicbox #play.pic3, 350, 100, 93, 93 'Add a control that will be used to announce the results of each play textbox #play.textbox1 130, 230, 240, 50 'Add a button control to operate the slot machine button #play.button1 "Spin", AnimateDisplay, UL, 200, 300, 100, 30 'Add a groupbox control at the bottom of the window groupbox #play.groupbox1 "Stats:", 60, 350, 380, 100 'Use statictext controls to display 2 labels inside the groupbox control statictext #play.statictext3, "No of Games Played:", 90, 372, 105, 20 statictext #play.statictext4, "Account:", 290, 372, 50, 20 'Add 2 textbox controls inside the groupbox control for displaying 'game statistics textbox #play.textbox2 90, 395, 100, 20 textbox #play.textbox3 290, 395, 100, 20 'Open the window with no frame and a handle of #play open "Slot Machine" for window_nf as #play 'Set up the trapclose event for the window print #play, "trapclose ClosePlay" 'Set the font type, size, and attributes print #play.statictext1, "!font Arial 24 bold" print #play.textbox1, "!font Arial 24 bold" 'Display the initial values representing the number of games played and 'the value of the player's account print #play.textbox3, str$(account) print #play.textbox2, str$(gamesPlayed) print #play.button1, "!setfocus"; 'Set focus to the button control 'Pause the application and wait for the player's instruction wait end sub 'This subroutine is responsible for controlling the timing involved in 'displaying the slot machine's animation sub AnimateDisplay handle$ timer 333, DisplayImages 'Call the DisplayImages subroutine every '.333 seconds end sub 'This subroutine displays a different set of bitmap image files in the 'game's 3 bmpcontrols each time it is called. sub DisplayImages iteration = iteration + 1 'Keep track of how many times this subroutine 'has been called 'Display a different set of bitmap images upon each call if iteration = 1 then call UpdateDisplay "Apple", "Cherry", "Pear" if iteration = 2 then call UpdateDisplay "Cherry", "Pear", "Cherry" if iteration = 3 then call UpdateDisplay "Pear", "Apple", "Pear" if iteration = 4 then call UpdateDisplay "Apple", "Pear", "Cherry" if iteration = 5 then call UpdateDisplay "Pear", "Cherry", "Apple" 'Turn the timer control off on the 6th iteration if iteration = 6 then timer 0 'Turn the timer off iteration = 0 'Reset this value to zero call RandomSelection 'Call the subroutine that generates the slot end if 'machine's 6th spin end sub 'This subroutine loads the specified bitmap files into the game's 3 'bmpbutton controls sub UpdateDisplay x$, y$, z$ print #play.pic1, "drawbmp " + x$ + " 1 1" 'Load first image print #play.pic2, "drawbmp " + y$ + " 1 1" 'Load second image print #play.pic3, "drawbmp " + z$ + " 1 1" 'Load third image 'Use the flush command to make sure the images stick print #play.pic1, "flush" print #play.pic2, "flush" print #play.pic3, "flush" 'Let's make a little noise at the end of each spin playwave "ding.wav", asynch end sub 'This subroutine is responsible for determining which bitmap images should 'be displayed for the slot machine's 6th and final spin sub RandomSelection RandomNumber = int(rnd(1)*3) + 1 'Retrieve a number between 1 and 3 'Select the image to be displayed on the first bmpbutton control SELECT CASE RandomNumber CASE 1 print #play.pic1, "drawbmp Cherry 1 1" 'Display the Cherry bitmap firstPic = 1 'Set a numeric value representing the selection CASE 2 print #play.pic1, "drawbmp Apple 1 1" 'Display the Apple bitmap firstPic = 2 'Set a numeric value representing the selection CASE 3 print #play.pic1, "drawbmp Pear 1 1" 'Display the Pear bitmap firstPic = 3 'Set a numeric value representing the selection END SELECT RandomNumber = int(rnd(1)*3) + 1 'Retrieve a number between 1 and 3 'Select the image to be displayed on the second bmpbutton control SELECT CASE RandomNumber CASE 1 print #play.pic2, "drawbmp Cherry 1 1" 'Display the Cherry bitmap secondPic = 1 'Set a numeric value representing the selection CASE 2 print #play.pic2, "drawbmp Apple 1 1" 'Display the Apple bitmap secondPic = 2 'Set a numeric value representing the selection CASE 3 print #play.pic2, "drawbmp Pear 1 1" 'Display the Pear bitmap secondPic = 3 'Set a numeric value representing the selection END SELECT RandomNumber = int(rnd(1)*3) + 1 'Retrieve a number between 1 and 3 'Select the image to be displayed on the third bmpbutton control SELECT CASE RandomNumber CASE 1 print #play.pic3, "drawbmp Cherry 1 1" 'Display the Cherry bitmap thirdPic = 1 'Set a numeric value representing the selection CASE 2 print #play.pic3, "drawbmp Apple 1 1" 'Display the Apple bitmap thirdPic = 2 'Set a numeric value representing the selection CASE 3 print #play.pic3, "drawbmp Pear 1 1" 'Display the Pear bitmap thirdPic = 3 'Set a numeric value representing the selection END SELECT 'Let's make a little noise and display the results of the game playwave "ding.wav", asynch 'Tabulate the value representing the results of the 6th spin result = firstPic + secondPic + thirdPic 'Look to see if 3 cherries or 3 pears were displayed if (result = 3) or (result = 9) then print #play.textbox1, "Jackpot!" account = account + 3 'Add 3 dollars to the player's account end if 'A value of 6 means either 3 apples were displayed or 3 separate 'values were displayed if result = 6 then if firstPic = secondPic then 'Look for three apples print #play.textbox1, "Jackpot!" account = account + 3 'Add 3 dollars to the player's account else 'A cherry, apple, and pear were displayed print #play.textbox1, "You lose!" account = account – 5 'Subtract 5 dollars from the player's account end if end if 'A value other than 3, 6, or 9 means that 2 of a kind was displayed if (result <> 3) and (result <> 6) and (result <> 9) then print #play.textbox1, "Two of a kind!" account = account + 1 'Add 1 dollar to the player's account end if if account < 0 then 'End the game if the player goes broke notice "You have gone broke. Game Over!" 'Tell the player first close #play 'Close the window end 'Terminate the game end if 'Keep track of the total number of games played gamesPlayed = gamesPlayed + 1 'Update the display of game statistics print #play.textbox2, str$(gamesPlayed) print #play.textbox3, str$(account) end sub 'This subroutine is called when the player closes the #play window 'and is responsible for ending the game sub ClosePlay handle$ 'Get confirmation before terminating program execution confirm "Are you sure you want quit?"; answer$ if answer$ = "yes" then 'The player clicked on Yes close #play 'Close the #play window end 'Terminate the game end if end sub
Collepals.com Plagiarism Free Papers
Are you looking for custom essay writing service or even dissertation writing services? Just request for our write my paper service, and we'll match you with the best essay writer in your subject! With an exceptional team of professional academic experts in a wide range of subjects, we can guarantee you an unrivaled quality of custom-written papers.
Get ZERO PLAGIARISM, HUMAN WRITTEN ESSAYS
Why Hire Collepals.com writers to do your paper?
Quality- We are experienced and have access to ample research materials.
We write plagiarism Free Content
Confidential- We never share or sell your personal information to third parties.
Support-Chat with us today! We are always waiting to answer all your questions.
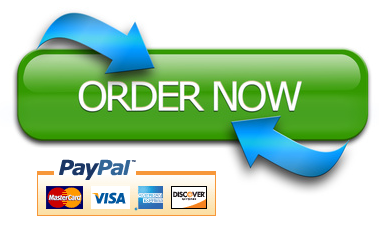