Develop a complete use case for software implementing a system for: searching for books on a specific topic using an online bookstore. > Write a user story
Develop a complete use case for software implementing a system for: searching for books on a specific topic using an online bookstore.
> Write a user story for the above software.
> Write a nonfunctional requirement for the application.
Outline your plan addressing these issues and other issues.
Need 6-8 pages APA-formatted paper with minimum of 9 peer-reviewed citations.
84
What is it? Software engineering practice is a broad array of principles, concepts, methods, and tools that you must consider as software is planned and developed. Principles that guide practice establish a foundation from which software engineering is conducted.
Who does it? Practitioners (software engineers) and their managers conduct a variety of soft- ware engineering tasks.
Why is it important? Software process pro- vides everyone involved in the creation of a computer-based system or product with a road map for getting to a successful destina- tion. Software practice provides you with the details you’ll need to drive along the road. It tells you where the bridges, the roadblocks, and the forks are located. It helps you under- stand the concepts and principles that must be understood and followed to drive safely and rapidly. It instructs you on how to drive, where to slow down, and where to speed up. In the context of software engineering, practice is what you do day in and day out as software evolves from an idea to a reality.
What are the steps? Four elements of practice apply regardless of the process model that is chosen. They are principles, concepts, meth- ods, and tools. Tools support the application of methods.
What is the work product? Practice encom- passes the technical activities that produce all work products that are defined by the soft- ware process model that has been chosen.
How do I ensure that I’ve done it right? First, have a firm understanding of the principles that apply to the work (e.g., design) that you’re doing at the moment. Then, be certain that you’ve chosen an appropriate method for the work, be sure that you understand how to apply the method, use automated tools when they’re appropriate for the task, and be adamant about the need for techniques to ensure the quality of work products that are produced. You also need to be agile enough to make changes to your plans and methods as needed.
Q u i c k L o o k
coding principles . . . . . . . . . . . . . . . . . . . . . . . 96 communication principles . . . . . . . . . . . . . . . . 88 core principles . . . . . . . . . . . . . . . . . . . . . . . . . 85 deployment principles . . . . . . . . . . . . . . . . . . 98 modeling principles . . . . . . . . . . . . . . . . . . . . . 92
planning principles . . . . . . . . . . . . . . . . . . . . . 91 practice . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 85 process . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 85 testing principles . . . . . . . . . . . . . . . . . . . . . . . 96
k e y c o n c e p t s
C H A P T E R
6 Software engineers are often depicted as working long hours by themselves to meet impossible deadlines without connecting to other people. This is a dark image of software engineering practice to be sure. Yet, as we discussed in the previous chapters, most software engineers work on teams and frequently interact with their stakeholders. If you search for surveys of technical professionals on the Internet, you will see software engineers listed among those experiencing the greatest satisfaction from their jobs.
Principles That Guide Practice
CHAPTER 6 PRINCIPLES THAT GUIDE PRACTICE 85
People who create computer software practice the art or craft or discipline1 that is software engineering. But what is software engineering “practice”? In a generic sense, practice is a collection of concepts, principles, methods, and tools that a software engineer calls upon on a daily basis. Practice allows managers to manage software projects and software engineers to build computer programs. Practice populates a software process model with the necessary technical and management how-to’s to get the job done. Practice transforms a haphazard unfocused approach into something that is more organized, more effective, and more likely to achieve success.
Various aspects of software engineering practice will be examined throughout the remainder of this book. In this chapter, our focus is on principles and concepts that guide software engineering practice in general.
6 .1 co r e pr i nc i p L e s
Software engineering is guided by a collection of core principles that help in the application of a meaningful software process and the execution of effective software engineering methods. At the process level, core principles establish a philosophical foundation that guides a software team as it performs the framework and umbrella activities and produces a set of software engineering work products. At the practice level, core principles establish a collection of values and rules that can guide you in analyzing a problem, designing a solution, implementing and testing the solution, and ultimately deploying the software so stakeholders can use it.
6.1.1 Principles That Guide Process In Part One of this book we discussed the importance of the software process and described several process models that have been proposed for software engineering work. Every project is unique, and every team is unique. That means you must adapt your process to best fit your needs. Regardless of the process model your team adopts, it contains elements of the generic process framework we described in Chapter 1. The following set of core principles can be applied to this framework and to every software process. A simplified view of this framework is shown in Figure 6.1.
Principle 1. Be agile. Whether the process model you choose is prescriptive or agile, the basic tenets of agile development should govern your approach. Every aspect of the work you do should emphasize economy of action—keep your techni- cal approach as simple as possible, keep the work products you produce as concise as possible, and make decisions locally whenever possible.
Principle 2. Focus on quality at every step. The exit condition for every process activity, action, and task should focus on the quality of the work product that has been produced.
Principle 3. Be ready to adapt. Process is not a religious experience, and dogma has no place in it. When necessary, adapt your approach to constraints imposed by the problem, the people, and the project itself.
1 Some writers argue for one of these terms to the exclusion of the others. In reality, software engineering is all three.
86 PART TWO MODELING
Principle 4. Build an effective team. Software engineering process and practice are important, but the bottom line is people. Build a self-organizing team that has mutual trust and respect.2
Principle 5. Establish mechanisms for communication and coordination. Projects fail because important information falls into the cracks and/or stakeholders fail to coordinate their efforts to create a successful end product. These are management issues, and they must be addressed.
Principle 6. Manage change. The approach may be either formal or informal, but mechanisms must be established to manage the way changes are requested, assessed, approved, and implemented.
Principle 7. Assess risk. Lots of things can go wrong as software is being developed. It’s essential that you establish contingency plans. Some of these contingency plans will form the basis for security engineering tasks (Chapter 18).
Principle 8. Create work products that provide value for others. Create only those work products that provide value for other process activities, actions, or tasks. Every work product that is produced as part of software engineering practice will be passed on to someone else. Be sure that the work product imparts the necessary information without ambiguity or omission.
Part Four of this book focuses on project and process management issues and considers various aspects of each of these principles in some detail.
6.1.2 Principles That Guide Practice Software engineering practice has a single overriding goal: to deliver on-time, high- quality, operational software that contains functions and features that meet the needs of all stakeholders. To achieve this goal, you should adopt a set of core principles that guide your technical work. These principles have merit regardless of the analysis
Communication Planning
Construction Deployment
Time
Modeling
Figure 6.1 Simplified process framework
2 The characteristics of effective software teams were discussed in Chapter 5.
CHAPTER 6 PRINCIPLES THAT GUIDE PRACTICE 87
and design methods that you apply, the construction techniques (e.g., programming language, automated tools) that you use, or the verification and validation approach that you choose. The following set of core principles is fundamental to the practice of software engineering:
Principle 1. Divide and conquer. Stated in a more technical manner, analysis and design should always emphasize separation of concerns (SoCs). A large problem is easier to solve if it is subdivided into a collection of elements (or concerns).
Principle 2. Understand the use of abstraction. At its core, an abstraction is a simplification of some complex element of a system used to communicate meaning in a single phrase. When we use the abstraction spreadsheet, it is assumed that you understand what a spreadsheet is, the general structure of content that a spreadsheet presents, and the typical functions that can be applied to it. In software engineering practice, you use many different levels of abstraction, each imparting or implying meaning that must be communicated. In analysis and design work, a software team normally begins with models that represent high levels of abstraction (e.g., a spreadsheet) and slowly refines those models into lower levels of abstraction (e.g., a column or the SUM function).
Principle 3. Strive for consistency. Whether it’s creating an analysis model, developing a software design, generating source code, or creating test cases, the principle of consistency suggests that a familiar context makes software easier to use. As an example, consider the design of a user interface for a mobile app. Consistent placement of menu options, the use of a consistent color scheme, and the consistent use of recognizable icons help to create a highly effective user experience.
Principle 4. Focus on the transfer of information. Software is about information transfer—from a database to an end user, from a legacy system to a WebApp, from an end user into a graphic user interface (GUI), from an operating system to an application, from one software component to another—the list is almost endless. In every case, information flows across an interface, and this means there are opportunities for errors, omissions, or ambiguity. The implication of this principle is that you must pay special attention to the analysis, design, construction, and testing of interfaces.
Principle 5. Build software that exhibits effective modularity. Separation of concerns (Principle 1) establishes a philosophy for software. Modularity provides a mechanism for realizing the philosophy. Any complex system can be divided into modules (components), but good software engineering practice demands more. Modularity must be effective. That is, each module should focus exclusively on one well-constrained aspect of the system. Additionally, modules should be interconnected in a relatively simple manner to other modules, to data sources, and to other environ- mental aspects.
Principle 6. Look for patterns. Software engineers use patterns as a means of cataloging and reusing solutions to problems they have encountered in the past. The use of these design patterns can be applied to wider systems engineering and systems integration problems, by allowing components in complex systems to evolve independently. Patterns will be discussed further in Chapter 14.
Principle 7. When possible, represent the problem and its solution from several different perspectives. When a problem and its solution are examined from different
88 PART TWO MODELING
perspectives, it is more likely that greater insight will be achieved and that errors and omissions will be uncovered. The unified modeling language (UML) provides a means of describing a problem solution from multiple viewpoints, as described in Appendix 1.
Principle 8. Remember that someone will maintain the software. Over the long term, software will be corrected as defects are uncovered, adapted as its environ- ment changes, and enhanced as stakeholders request more capabilities. These main- tenance activities can be facilitated if solid software engineering practice is applied throughout the software process.
These principles are not all you’ll need to build high-quality software, but they do establish a foundation for every software engineering method discussed in this book.
6.2 principLes that Guide each Framework activity
In the sections that follow, we consider principles that have a strong bearing on the success of each generic framework activity defined as part of the software process. In many cases, the principles that are discussed for each of the framework activities are a refinement of the principles presented in Section 6.1. They are simply core principles stated at a lower level of abstraction.
6.2.1 Communication Principles Before customer requirements can be analyzed, modeled, or specified, they must be gathered through the communication activity. A customer has a problem that may be amenable to a computer-based solution. You respond to the customer’s request for help. Communication has begun. But the road from communication to understanding is often full of potholes.
Effective communication (among technical peers, with the customer and other stakeholders, and with project managers) is among the most challenging activities that you will confront. There are many ways to communicate, but it’s important to recog- nize that not all are equal in richness or effectiveness (Figure 6.2). In this context, we discuss communication principles as they apply to customer communication. However, many of the principles apply equally to all forms of communication that occur within a software project.
The Difference Between Customers and End Users A customer is the person or group who
(1) originally requested the software to be built, (2) defines overall business objectives for the software, (3) provides basic product requirements, and (4) coordinates funding for the project. In a product or system business, the customer is often the marketing group. In an information technology
(IT) environment, the customer might be a business component or department.
An end user is the person or group who (1) will actually use the software that is built to achieve some business purpose and (2) will define opera- tional details of the software so the business purpose can be achieved. In some cases, the customer and the end user may be one and the same, but for many projects that is not the case.
inFo
CHAPTER 6 PRINCIPLES THAT GUIDE PRACTICE 89
Principle 1. Listen. Before communicating, be sure you understand the point of view of the other party, know a bit about his or her needs, and then listen. Try to focus on the speaker’s words, rather than formulating your response to those words. Ask for clarification if something is unclear, and avoid constant interruptions. Never become contentious in your words or actions (e.g., rolling your eyes or shaking your head) as a person is talking.
Principle 2. Prepare before you communicate. Spend the time to understand the problem before you meet with others. If necessary, do some research to understand business domain jargon. If you have responsibility for conducting a meeting, prepare an agenda in advance of the meeting.
Principle 3. Someone should facilitate the activity. Every communication meeting should have a leader (a facilitator) to keep the conversation moving in a productive direction, (2) to mediate any conflict that does occur, and (3) to ensure that other principles are followed.
Principle 4. Face-to-face communication is best. However, this communication usually works better when some other representation of the relevant information is present. For example, a participant may create a drawing or a “strawman” document that serves as a focus for discussion.
Principle 5. Take notes and document decisions. Things have a way of falling into the cracks. Someone participating in the communication should serve as a “recorder” and write down all important points and decisions.
Principle 6. Strive for collaboration. Collaboration and consensus occur when the collective knowledge of members of the team is used to describe product or system functions or features. Each small collaboration serves to build trust among team members and creates a common goal for the team.
E-mail Text
Telephone
Video-conference
Face-to-face
Richness of Communication
C om
m un
ic at
io n
E� ec
tiv en
es s
Paper
Figure 6.2 Effectiveness of communication modes
90 PART TWO MODELING
Principle 7. Stay focused; modularize your discussion. The more people are involved in any communication, the more likely that discussion will bounce from one topic to the next. The facilitator should keep the conversation modular, leaving one topic only after it has been resolved (however, see Principle 9).
Principle 8. If something is unclear, draw a picture. Verbal communication goes only so far. A sketch or drawing can often provide clarity when words fail to do the job.
Principle 9. (a) Once you agree to something, move on. (b) If you can’t agree to something, move on. (c) If a feature or function is unclear and cannot be clarified right now, move on. Communication, like any software engineering activity, takes time. Rather than iterating endlessly, the people who participate should recognize that many topics require discussion (see Principle 2) and that “moving on” is sometimes the best way to achieve communication agility.
Principle 10. Negotiation is not a contest or a game. It works best when both parties win. There are many instances in which you and other stakeholders must negotiate functions and features, priorities, and delivery dates. If the team has collaborated well, all parties have a common goal. Still, negotiation will demand compromise from all parties.
Communication Mistakes
The scene: Software engineer- ing team workspace
The players: Jamie Lazar, software team member; Vinod Raman, software team member; Ed Robbins, software team member.
The conversation: Ed: What have you heard about this SafeHome project?
Vinod: The kickoff meeting is scheduled for next week.
Jamie: I’ve already done a little bit of investi- gation, but it didn’t go well.
Ed: What do you mean?
Jamie: Well, I gave Lisa Perez a call. She’s the marketing honcho on this thing.
Vinod: And . . . ?
Jamie: I wanted her to tell me about SafeHome features and functions . . . that sort of thing. Instead, she began asking me questions about security systems, surveillance systems . . . I’m no expert.
Vinod: What does that tell you?
(Jamie shrugs)
Vinod: That marketing will need us to act as consultants and that we’d better do some homework on this product area before our kickoff meeting. Doug said that he wanted us to “collaborate” with our customer, so we’d better learn how to do that.
Ed: Probably would have been better to stop by her office. Phone calls just don’t work as well for this sort of thing.
Jamie: You’re both right. We’ve got to get our act together or our early communications will be a struggle.
Vinod: I saw Doug reading a book on “requirements engineering.” I’ll bet that lists some principles of good communication. I’m going to borrow it from him.
Jamie: Good idea . . . then you can teach us.
Vinod (smiling): Yeah, right.
saFehome
CHAPTER 6 PRINCIPLES THAT GUIDE PRACTICE 91
6.2.2 Planning Principles The planning activity encompasses a set of management and technical practices that enable the software team to define a road map as it travels toward its strategic goal and tactical objectives.
Try as we might, it’s impossible to predict exactly how a software project will evolve. There is no easy way to determine what unforeseen technical problems will be encountered, what important information will remain undiscovered until late in the project, what misunderstandings will occur, or what business issues will change. And yet, a good software team must plan its approach. Often planning is iterative (Figure 6.3).
There are different planning philosophies.3 Some people are “minimalists,” arguing that change often obviates the need for a detailed plan. Others are “traditionalists,” arguing that the plan provides an effective road map and the more detail it has, the less likely the team will become lost.
What to do? On many projects, overplanning is time consuming and fruitless (too many things change), but underplanning is a recipe for chaos. Like most things in life, planning should be agile and conducted in moderation, enough to provide useful guid- ance for the team—no more, no less. Regardless of the rigor with which planning is conducted, the following principles always apply:
Principle 1. Understand the scope of the project. It’s impossible to use a road map if you don’t know where you’re going. Scope provides the software team with a destination.
Principle 2. Involve stakeholders in the planning activity. Stakeholders define priorities and establish project constraints. To accommodate these realities, software engineers must often negotiate order of delivery, time lines, and other project- related issues.
3 A detailed discussion of software project planning and management is presented in Part Four of this book.
Initial risks and project scope
Identify the highest risks
Plan and develop
Assess
Iteration N
Risk eliminated
Revise risk assessment
Revise project plan
Figure 6.3 Iterative planning
92 PART TWO MODELING
Principle 3. Recognize that planning is iterative. A project plan is never engraved in stone. As work begins, it is very likely that things will change. The plan will need to be adjusted. Iterative, incremental process models include time for revising plans after the delivery of each software increment based on feedback received from users.
Principle 4. Estimate based on what you know. The intent of estimation is to provide an indication of effort, cost, and task duration, based on the team’s current understanding of the work to be done. If information is vague or unreliable, estimates will be equally unreliable.
Principle 5. Consider risk as you define the plan. If you have identified risks that have high impact and high probability, contingency planning is necessary. The project plan (including the schedule) should be adjusted to accommodate the likelihood that one or more of these risks will occur.
Principle 6. Be realistic. People don’t work 100 percent of every day. Changes will occur. Even the best software engineers make mistakes. These and other realities should be considered as a project plan is established.
Principle 7. Adjust granularity as you define the plan. The term granularity refers to the detail with which some element of planning is represented or conducted. A high-granularity plan provides significant work task detail that is planned over relatively short time increments (so that tracking and control occur frequently). A low-granularity plan provides broader work tasks that are planned over longer time periods. In general, granularity moves from high to low as the project time line moves away from the current date. Activities that won’t occur for many months do not require high granularity (too much can change).
Principle 8. Define how you intend to ensure quality. The plan should identify how the software team intends to ensure quality. If technical reviews4 are to be conducted, they should be scheduled. If pair programming (Chapter 3) is to be used during construction, it should be explicitly defined within the plan.
Principle 9. Describe how you intend to accommodate change. Uncontrolled change can obviate even the best planning. You should identify how changes are to be accommodated as software engineering work proceeds. For example, can the customer request a change at any time? If a change is requested, is the team obliged to implement it immediately? How is the impact and cost of the change assessed?
Principle 10. Track the plan frequently, and make adjustments as required. Software projects fall behind schedule one day at a time. Therefore, it makes sense to track progress daily, looking for problem areas and situations in which scheduled work does not conform to actual work conducted. When slippage is encountered, the plan is adjusted accordingly.
To be most effective, everyone on the software team should participate in the planning activity. Only then will team members “sign up” to the plan.
6.2.3 Modeling Principles We create models to gain a better understanding of the actual entity to be built. When the entity is a physical thing (e.g., a building, a plane, a machine), we can build a
4 Technical reviews are discussed in Chapter 16.
CHAPTER 6 PRINCIPLES THAT GUIDE PRACTICE 93
three-dimensional (3D) model that is identical in form and shape but smaller in scale. However, when the entity to be built is software, our model must take a different form. It must be capable of representing the information that software transforms, the architecture and functions that enable the transformation to occur, the features that users desire, and the behavior of the system as the transformation is taking place. Models must accomplish these objectives at different levels of abstraction—first depicting the software from the customer’s viewpoint and later representing the soft- ware at a more technical level. Figure 6.4 shows how modeling may be used in agile software design.
In software engineering work, two classes of models can be created: requirements models and design models. Requirements models (also called analysis models) repre- sent customer requirements by depicting the software in three different domains: the information domain, the functional domain, and the behavioral domain (Chapter 8). Design models represent characteristics of the software that help practitioners to con- struct it effectively: the architecture, the user interface, and component-level detail (Chapters 9 through 12).
In their book on agile modeling, Scott Ambler and Ron Jeffries [Amb02] define a set of modeling principles5 that are intended for those who use an agile process model (Chapter 3) but are appropriate for all software engineers who perform modeling action and tasks:
Principle 1. The primary goal of the software team is to build software, not create models. Agility means getting software to the customer in the fastest possible time. Models that make this happen are worth creating, but models that slow the process down or provide little new insight should be avoided.
Modeling Quick Design
Quick Design
Deployment, Delivery, Feedback
Communication
Construction of Prototype
Figure 6.4 Role of software modeling
5 The principles noted in this section have been abbreviated and rephrased for the purposes of this book.
94 PART TWO MODELING
Principle 2. Travel light—don’t create more models than you need. Every model that is created must be kept up to date as changes occur. More importantly, every new model takes time that might otherwise be spent on construction (coding and testing). Therefore, create only those models that make it easier and faster to construct the software.
Principle 3. Strive to produce the simplest model that will describe the prob- lem or the software. Don’t overbuild the software [Amb02]. By keeping models simple, the resultant software will also be simple. The result is software that is easier to integrate, easier to test, and easier to maintain (to change). In addition, simple models are easier for members of the software team to understand and critique, resulting in an ongoing for
Collepals.com Plagiarism Free Papers
Are you looking for custom essay writing service or even dissertation writing services? Just request for our write my paper service, and we'll match you with the best essay writer in your subject! With an exceptional team of professional academic experts in a wide range of subjects, we can guarantee you an unrivaled quality of custom-written papers.
Get ZERO PLAGIARISM, HUMAN WRITTEN ESSAYS
Why Hire Collepals.com writers to do your paper?
Quality- We are experienced and have access to ample research materials.
We write plagiarism Free Content
Confidential- We never share or sell your personal information to third parties.
Support-Chat with us today! We are always waiting to answer all your questions.
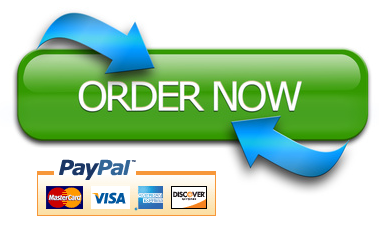