WeatherData
Create a class called WeatherData that will store information about daily weather conditions for NASA. It should be able to store the current daily temperature as well as wind speed. You also want to use good object-oriented programming (OOP) practices and ensure that the WeatherData class contains the following methods:
Default constructor: WeatherData()
Overloaded constructor: WeatherData(float dailyTemp, int windSpeed)
Mutators / set methods: void setDailyTemp(float newTemp), void setWindSpeed(int newWindSpeed)
Accessors / get methods: float getDailyTemp(), int getWindSpeed()
String method that returns a description of all class data as a string: String toString()
Equals method that can compare WeatherData objects: boolean equals(Object obj)
Ensure that the WeatherData class is stored in a file called WeatherData.java. Then create another class called Test (within the same project) that contains the main() method with the following test code:
WeatherData day1 = new WeatherData();
// Next two lines will test the set() methods
day1.setDailyTemp(75.0);
day1.setWindSpeed(100);
// This next line tests the toString() method in WeatherData for Day1
System.out.println(“Day1->” + day1);
WeatherData day2 = new WeatherData(52.0, 10);
// This next line tests the toString() method in WeatherData for Day2
System.out.println(“Day2->” + day2);
// This next line will test the equals() method in WeatherData with two different objects
if(day1 == day2)
System.out.println(“Day1 is equal to Day2”);
else
System.out.println(“Day1 is NOT equal to Day2”);
// This next line will test the equals() method in WeatherData with two equal objects
WeatherData day3 = new WeatherData(75.0, 100);
if(day1 == day3)
System.out.println(“Day1 is equal to Day3”);
else
System.out.println(“Day1 is NOT equal to Day3”);
// The next two lines will test the get() methods of the WeatherData class.
System.out.println(“Day2 Daily Temperature: “ + day2.getDailyTemp());
System.out.println(“Day3 Wind Speed: “ + day3.getWindSpeed());
Ensure that the test class is stored in a file called Test.java. When you run the Test application, the output should be as follows:
Day1->Weather Data: Daily Temperature = 75.0 F. Wind Speed = 100 mph.
Day2->Weather Data: Daily Temperature = 52.0 F. Wind Speed = 10 mph.
Day1 is NOT equal to Day2
Day1 is equal to Day3
Day2 Daily Temperature: 52.0
Day3 WindSpeed: 100
Collepals.com Plagiarism Free Papers
Are you looking for custom essay writing service or even dissertation writing services? Just request for our write my paper service, and we'll match you with the best essay writer in your subject! With an exceptional team of professional academic experts in a wide range of subjects, we can guarantee you an unrivaled quality of custom-written papers.
Get ZERO PLAGIARISM, HUMAN WRITTEN ESSAYS
Why Hire Collepals.com writers to do your paper?
Quality- We are experienced and have access to ample research materials.
We write plagiarism Free Content
Confidential- We never share or sell your personal information to third parties.
Support-Chat with us today! We are always waiting to answer all your questions.
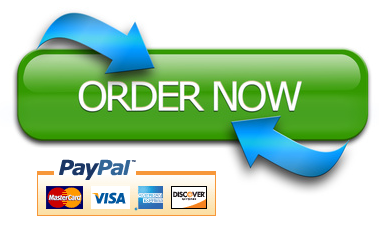