University of Central Florida tip calculator code
Assignment 10: Tip Calculator App For this assignment, you will create a Tip Calculator App that uses a form to take in multiple types of user input. Requirements: • Create a new iOS app project called TipCalculator using Swift and SwiftUI. This app will be a tip calculator intended for use at a restaurant when someone wants to calculate a gratuity (tip) amount based on a specific percentage of the overall bill for a meal. In addition to calculating the tip amount, the app will also add the tip to the bill amount to show the user the entire amount to provide to the wait staff (bill + tip). Further, the app will allow for splitting the bill, and will calculate the amount due per person if more than one person is selected. • First, use SwiftUI to create a form with four sections inside of it. The sections should be labeled with Section headers, as follows: • • • • Check Amount Number of People Splitting the Check Tip Percentage Amount Owed Per Person • Declare four @State variables, as follows, and set each to a default value of your choice: • checkAmount (double) • numberOfPeople (integer) • tipPercentage (integer) • Declare one @FocusState variable • amountIsFocused (Boolean) • Declare one array of integers: • tipPercentages (array of integers) – set to include the following values: 0, 10, 15, 20, 25 • Declare one computed property: • • totalPerPerson (double) Inside the computed property use the following logic statements: i. let peopleCount = Double(numberOfPeople + 1) ii. let tipSelection = Double(tipPercentage) iii. let tipValue = tipSelection / 100 iv. let grandTotal = checkAmount + (checkAmount * tipValue) v. let amountPerPerson = grandTotal / peopleCount vi. return amountPerPerson • In the Check Amount section, include a TextField: • With the value set to the checkAmount State variable, using two-way binding • That uses an appropriate Keyboard Type • That has the .focused() modifier, set to the amountIsFocused State variable, using two-way binding • In the Number of People Splitting the Check section, include a Picker: • With the value set to the numberOfPeople State Variable, using two-way binding • Using an appropriate PickerStyle (wheel, etc.) • That uses a ForEach loop to loop from 1 to 10 and displays the loop control variable (remember this will be “($0)” when using a ForEach loop in a Text field • In the Tip Percentage section, include a Picker: • With the value set to the tipPercentage State Variable, using two-way binding • That uses the .segmented PickerStyle • That uses a ForEach loop to loop through the tipPercentages array and displays the loop control variable (remember this will be “($0)” when using a ForEach loop in a Text field i. Hint: you may need the id: .self for this one • In the Amount Owed Per Person section, include a Text field: • With the value set to the totalPerPerson State Variable • Make sure to display in currency format (USD) • Enclose the entire form inside a NavigationView • Add a .toolbar modifier to the Form • The toolbar should include a button called “Done” • When the done button is clicked, change the amountIsFocused variable to false • Save and test your app to make sure that all functionality is working properly. • Submit a .zip file containing your Xcode project folder (outer folder).
Collepals.com Plagiarism Free Papers
Are you looking for custom essay writing service or even dissertation writing services? Just request for our write my paper service, and we'll match you with the best essay writer in your subject! With an exceptional team of professional academic experts in a wide range of subjects, we can guarantee you an unrivaled quality of custom-written papers.
Get ZERO PLAGIARISM, HUMAN WRITTEN ESSAYS
Why Hire Collepals.com writers to do your paper?
Quality- We are experienced and have access to ample research materials.
We write plagiarism Free Content
Confidential- We never share or sell your personal information to third parties.
Support-Chat with us today! We are always waiting to answer all your questions.
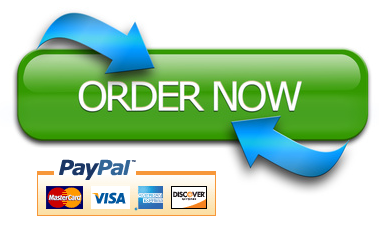