Programming Question
Topic: • Enhance the prototype created in Assignment 1 with jQuery validation. This version will be used in the next assignments. Before you begin: • • • • • • • • You must complete Assignment 1 before proceeding. You must know how to search and use the online jQuery Mobile Docs to enhance your mobile app. Make yourself familiar with the hands-on examples done in the lab during class time. Make sure to view the screenshots in color, as you will be required to match the color themes used exactly. The assignment specification and screenshots are made assuming a student’s name Jason Bourne (and initial is JB). Replace Jason Bourne with your full name, JB with your own initial. Also replace xx and XX with your own initials. Applying proper initials will be treated as programming standards. All javascript files must have your initial as prefix. See AvengersDB-iteration2 hands on program in details. The screenshots below are taken from Chrome browser without using any device skin. Problem Specification: Task 1: Create Assignment 2 project • Create an empty project and name it: XXFeedbackA2 (where XX is your initials). Copy all the files and folders from your assignment 1 project except the folder called .idea (.idea is a hidden folder created by WebStorm. If you use any other IDE, the .idea folder will not exist). • Add the following Javascript files under js folder: o xxdatabase.js o xxDAL.js o xxfacade.js o xxutil.js o xxglobal.js • Add the following Stylesheet file under css folder: o xxmystyle.css • Include all of them to index.html. • Add ready event to xxglobal.js file. All the event handlers must be defined in xxglobal.js file. • Provide name and id attributes for the following items in your project. Missing id and name will result in deduction of marks (Check the marking sheet – the last page of the document). These requirements will be considered as Standards in this course. List/Combobox Textarea Checkbox Button √ √ √ √ √ √ Radiobutton √ √ √ √ Table 1: id and name requirement chart Task 2: Update ‘Home’ page Update the headers to indicate Assignment 2 (Replace A1 with A2). Also replace other references to A1 with A2 in the page content. See the screenshot below. Rest of the home page will be the same as your assignment 1. Figure 1: Screenshot of Home page for Assignment 2 Task 3: Update ‘Add Feedback’ page • • • Update the page according to the following screenshot. Add a “Type” ComboBox containing “Other”, “Asian” and “Canadian”. “Other” will be shown by default. Please check Figure 2. If ‘Do you want to add your ratings’ checkbox is checked then 4 rating TextBoxes (Food Quality, Service, Value (Hint: input type=”number”) and Overall Rating (Calculated) (Hint: input type=”text”) will be visible. Otherwise, they will be hidden. Check Figure 3 and Figure 4. • • • • When the page is shown, ‘Do you want to add your ratings’ checkbox will be unchecked by default. Check Figure 3 Overall Rating textbox is Read-only. The 3 rating textboxes will be initialized with 0 whenever they become visible. (note: hide and show will reset the textboxes to 0 as well) Check Figure 5. Any change in the 3 rating text boxes will automatically update the overall Rating (calculated) Textbox (Show average will follow the formula: o (quality + service + value) * 100/15 . o Add a % symbol. o Check Figure 4 Implement the show/hide feature in xxglobal.js file. Hint: put the 4 Textboxes in a div, show and hide the div if the check box is checked/unchecked, respectively. See jQuery built in function show(), hide() (https://www.w3schools.com/jquery/jquery_hide_show.asp) Figure 2: ComboBox content Figure 3: Screenshot of Add Feedback page – Do you want to add your ratings unchecked. Figure 4: Screenshot of Add Feedback page – Do you want to add your ratings checked. Figure 5: Ratings are initialized with 0 when they become visible (by selecting Do you want to add your ratings checkbox) Task 4: Update ‘Modify Feedback’ Page • • Update the ‘Modify Feedback’ page in the same way as ‘Add Feedback’ page. ‘Do you want to add your ratings’ checkbox will behave in the same way. Check the following screenshot(s) Figure 6 and Figure 7 Figure 6: Screenshot of Modify Feedback page – Do you want to add your ratings unchecked. Figure 7: Screenshot of Modify Feedback page – Do you want to add your ratings checked. Task 5: Implement validation • • • • Include jQuery validation Javascript (available in_lib.zip) to the index.html file. your validation logic in xxutil.js file. Add the following styles to xxmystyle.css file: label.error{ color: #F00; fontweight: bold; } input.error, select.error, textarea.error { border: 1px red solid; } Define Both ‘Add a Feedback’ and ‘Modify Feedback’ pages will have similar validation (defined in xxutil.js file). Hint: You need to implement 2 validation functions; one for each form. However, it is also possible to make a parameterized validation function accepting references to the form. The validation will get triggered and show necessary messages below input fields when: o Save button is clicked on Add Feedback page. Print “Add Form is valid” if all inputs are valid and “Add Form is invalid” if there are invalid inputs. o Update button is clicked on Modify Feedback page. Print “Modify Form is valid” if all inputs are valid and “Modify Form is invalid” if there are invalid inputs. • Validation rules: o You may specify your own error message string. o Business Name: Required and length must be 2-20 characters long. o Reviewer Email: Required and valid email address Any valid email will be sufficient. Making only Conestoga email valid will result in deduction of marks. o Review Date: Required o If ‘Do you want to add your ratings?’ checkbox is checked o Food Quality, Service and Value: Value must be 0-5 Note: don’t use required, min and max attributes for input controls in the html file, rather define your own rules and messages for them in xxutil.js file. I should be able to input any number, valid or invalid. The validation will show error message in case of invalid input. In case of invalid input in any of the ratings, Overall Rating (calculated) will be updated according to the usual formula mentioned in Task 3. No special checking is necessary. o Check the following screen shot carefully. Figure 8: Example Validation 1 – error message shown when “Save” button is clicked on Add Feedback page. Note console window beside the form. Also note how the Overall Rating is calculated. Figure 9: Example Validation 2 – error message shown when “Update” button is clicked on Modify Feedback page. Note console window beside the form. Figure 10: Example Validation 3 – no error message shown when “Update” button is clicked on Modify Feedback page with valid input. Note console window beside the form. Task 6: Update Settings page • • Settings page will show up with “Default Reviewer Email” filled with your own email address. Check Figure 11. Use your own Conestoga email address as initial email when the page is shown. ‘Save Defaults’ button will save the ‘Default reviewer Email’ to local storage and show a message through alert. Check Figure 12. Figure 11: Setting page (Default reviewer email is auto filled with your own email address) Figure 12: Alert shown when Save Defaults is clicked. Figure 13: Screen shot of Chrome Developers Tools (Application tab) showing Local Storage Task 7: Test your app • • Open Developer Tool of chrome. You may use normal browser mode to test your application. Check if your application pages look exactly the same as screen shots provided in this specification document. • Check if your application behaves according to this specification. Task 8: Upload app • Make a zip file xxAssignment2.zip that will contain: o Complete project for your app o After unzipping, I should be able to run your application without any modification. Marking Sheet Description Marks Allocated 1 Home Page designed as specified 10 2 Add a Feedback page is designed as specified 10 3 Add a Feedback page ratings show/hide 10 4 Add a Feedback page validation (in Save button’s click 15 handler) 5 Modify Feedback page is designed as specified 10 6 Modify Feedback page ratings show/hide 5 7 Modify Feedback page validation (in Update button’s click handler) 10 8 Settings page stores info to local storage 10 9 Overall rating is updated automatically whenever any 20 of the quality, service, value changes in add and modify pages. Deduction Runtime errors 15 x_______ Assignment Standard (proper project name, etc., see the 5 x ________ standard documents for detail) Programming Standard 1 x ________ Late Submission (softcopy) _________days id attribute is missing for any input control 3 x ________ name attribute is missing for any input control 3 x ________ JavaScript file name not prefixed 2 x ________ Making only Conestoga Email address valid 5 Using required, min, max attributes instead of defining your own rules and messages(each) 5 x _________ Bugs and other requirements mentioned in this specification 3-10 based on severity Failing to answer to questions during demo 5-10 based on severity Marks Achieved Total
Collepals.com Plagiarism Free Papers
Are you looking for custom essay writing service or even dissertation writing services? Just request for our write my paper service, and we'll match you with the best essay writer in your subject! With an exceptional team of professional academic experts in a wide range of subjects, we can guarantee you an unrivaled quality of custom-written papers.
Get ZERO PLAGIARISM, HUMAN WRITTEN ESSAYS
Why Hire Collepals.com writers to do your paper?
Quality- We are experienced and have access to ample research materials.
We write plagiarism Free Content
Confidential- We never share or sell your personal information to third parties.
Support-Chat with us today! We are always waiting to answer all your questions.
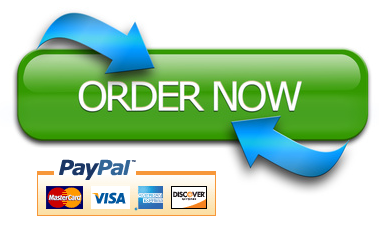