Grade changer
Objective
This assignment will consist of writing a program that gives practice with basic selection statements (if,else,switch), as well as further practice with basic I/O, and output formatting. As a real world application, this program will help you calculate your overall course grade at the end of the class if done correctly 🙂
Task
- Put the following const int variable declarations and initializations at the beginning of main()
const int ASSIGNPTSPOSS = 700;
const int MIDTERMPTSPOSS = 100;
const int REVELPTSPOSS = 500;
const int FINALPTSPOSS = 100;Note: these “Points Possible” totals are estimations that we’ll use for this assignment, and may not actually reflect the number of points possible in our course. You can modify these values later in the term (when we know more clearly the possible point total in each grade category) to accurately calculate your class grade.
- Declare any other variables you may use throughout your program at the beginning of main() and not throughout your code. Remember, you can always declare a variable at the beginning of the program and then initialize it LATER on.
- Ask the user for the following information in order:
- The total number of programming assignment points earned
- Midterm Score
- The total number of Pearson points earned
- Score on the final exam
- Note that this part will feel a little tedious with a lot of cout, cin, cout, cin, but it’s necessary here.
- Then, ask the user if they earned any extra credit. They should enter y for yes, or n for no. Allow BOTH UPPERCASE and LOWERCASE Y/y or N/n here.
- If the user enters an invalid character, the program should naturally end.
- If the user enters a valid character
- If they entered ‘y’ or ‘Y’ for yes, ask the user how many extra credit points they’ve earned (you may assume they will enter an int here) and add it to their overall homework points made.
- If they entered ‘n’ or ‘N’ for no, don’t prompt user for extra credit points and no extra credit points are added to the overall homework points.
- Calculate and display the user’s final course percentage.
- Anytime you print these values, make sure they print to the screen to one decimal place precision.
- The following formulas should help with your calculations: A = ((revelptsmade/REVELPTSPOSS) * .1)
B = (((assignptsmade+extracredit)/ASSIGNPTSPOSS) * .4)
C = ((midtermptsmade/MIDTERMPTSPOSS) * .2)
D = ((finalptsmade/FINALPTSPOSS) * .3)…and then:
(A+B+C+D) * 100 = The Final Course Grade
- Then, use either a switch statement or an if/else if block to determine what letter grade the user earned based on their final course grade percentage. See the course syllabus for the letter grade breakdowns. You don’t have to handle + or – letter grades, but you can if you choose. Just printing the basic letter grade is fine.
- Error Checking: You may ASSUME that the user will enter valid numerical grades, however, you need to check for an invalid character entry on their extra credit input. If the user enters something other than Y, y, N, n, print an error message (See sample run 3) and do not continue on with the rest of the displaying of the grades. Just allow the program to end naturally (meaning, structure your code so that the program control flow naturally makes its way to return 0; at the end of main()).
- Extra Credit Opportunity: Error check the numerical value entries. If the user entered an integer value < 0 for one or more of the numerical grade entries, do not proceed to ask about extra credit, print an error message, and allow the program to exit. You do not need to account for the instance in which the user enters the wrong data type.
Sample Runs
Below are some sample runs so you can see how your program should behave. Your formatting should match mine, EXACTLY. This means, spacing, prompts, wording, everything. (user input is underlined, to distinguish it from output)
Remember, the Underlined values show when USER ENTRY occurs, via cin.
SAMPLE RUN 1
Assignment Points: 700
Midterm Exam: 100
Revel Points: 500
Final Exam: 100
Did you earn extra credit? (y/n): n
Final Course Average: 100.0%
Final Course Letter Grade: A
SAMPLE RUN 2
Assignment Points: 650
Midterm Exam: 88
Revel Points: 442
Final Exam: 85
Did you earn extra credit? (y/n): y
Extra Credit Points Earned: 10
Final Course Average: 89.7%
Final Course Letter Grade: B
SAMPLE RUN 3
Assignment Points: 555
Midterm Exam: 76
Revel Points: 475
Final Exam: 60
Did you earn extra credit? (y/n): n
Final Course Average: 74.4%
Final Course Letter Grade: C
SAMPLE RUN 4
Assignment Points: 700
Midterm Exam: 100
Revel Points: 500
Final Exam: 100
Did you earn extra credit? (y/n): X
Invalid option, exiting program.
SAMPLE RUN 5 ( SHOWS EXTRA CREDIT OPTION )
Assignment Points: -50
Midterm Exam: 100
Revel Points: 500
Final Exam: 100
Invalid grade entry, exiting program.
Collepals.com Plagiarism Free Papers
Are you looking for custom essay writing service or even dissertation writing services? Just request for our write my paper service, and we'll match you with the best essay writer in your subject! With an exceptional team of professional academic experts in a wide range of subjects, we can guarantee you an unrivaled quality of custom-written papers.
Get ZERO PLAGIARISM, HUMAN WRITTEN ESSAYS
Why Hire Collepals.com writers to do your paper?
Quality- We are experienced and have access to ample research materials.
We write plagiarism Free Content
Confidential- We never share or sell your personal information to third parties.
Support-Chat with us today! We are always waiting to answer all your questions.
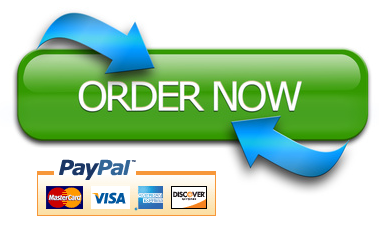