Rewrite the Programming Example in chapter 4 ( Cable Company Billing ). Expected Program and Design: 1. Write a pseudo code before starting your program ( you may n
Rewrite the Programming Example in chapter 4 ( Cable Company Billing ).
Expected Program and Design:
1. Write a pseudo code before starting your program ( you may not use SWITCH, replace it with IF structures )
1.1 Draw a flowchart for your program based on your pseudo code
2. Identify your constants
3. Your input will come from a text file of at least 15 customers
3.1 Input file format – customerType accountNumber premiumChannels
( i.e residential example: R12345 5 , business example B12345 16 8 )
residential customers
RaccountNumber numOfPremChannels
business customers
BaccountNumber numOfBasicServConn numOfPremChannels
4. Precision should be two decimal places
5. Calculate the running average for residential and business customer spending
6. Print all customer's bill to a single file and the end of the file you should have the average summary for each customer type.
6.1 Pay attention to details when you formatting your output
Note: Use all chapter concepts and make your final as a true representation of what we have learned this semester.
7. Implement your program using arrays, covered in chapter 8, and user defined simple data types to hold customer data.
Turn in
program design ( pseudo code and/or flow chart )
input file
output file
.cpp file of your program ( make sure you include your header and comment your code )
Transcript
Programming Example: Cable Company Billing
INTRODUCTION
This is a programming example for cable company billing.
A cable company has two types of customers: residential and business.
Residential customers pay a bill processing fee, a fee for basic services, and a per-channel fee for Premium channels.
Business customers pay a higher amount for bill processing and Premium channels as compared to residential customers.
Their basic service fee is also split into two components – a fixed amount for the first ten connections, and a fee for every additional connection.
PROBLEM ANALYSIS
You must write a program to calculate and print a customer's bill.
The program should ask for the customer's account number, customer code, number of premium channels to which the user subscribes, and, in the case of business customers, number of basic service connections.
PROGRAM OUTPUT
It should print the customer's account number and the billing amount.
To calculate the billing amount, you need to know the type of customer for whom the billing amount is calculated and the number of premium channels to which the customer has subscribed.
In the case of a business customer, you also need to know the number of basic service connections.
Other data needed to calculate the bill, such as the bill processing fees and the cost of a premium channel, are known quantities.
THE ALGORITHM
The problem analysis translates into the following algorithm.
First, set the precision to two decimal places.
Then, prompt the user for the account number and customer type.
Based on the customer type, determine the number of premium channels and basic service connections, compute the bill, and print the bill.
For residential customers, prompt the user for number of premium channels, compute the bill, and print the bill.
In the case of a business customer, prompt the user for the number of basic service connections, number of premium channels, compute the bill and print the bill.
COMPLETE PROGRAM LISTING
Based on the preceding discussion, you can now write the main program.
To output floating-point numbers in a fixed decimal format with two decimal places, the program must include the header file iomanip.
The cost of a basic service connection, and the cost of a premium channel are fixed, and these values are needed to compute the bill. Although these values are constants in the program, the cable company can change them with little warning.
To simplify the process of modifying the program later, instead of using these values directly in the program, you should declare them as named constants.
You need variables to store information such as the customer account number, customer code, number of premium channels, and number of basic service connections.
You also need a variable to store the calculated billing amount.
To output floating-point numbers with two decimal places, you must set the precision to two decimal places.
You must prompt the user to enter the account number and customer type.
These inputs must be stored in variables account number and customer type respectively.
The program uses a switch statement to calculate the bill for the desired customer.
For residential customers, you must prompt the user to enter the number of premium channels and stores the input in the variable number of premium channels.
The program uses a number of formulas to compute the billing amount. To compute the residential bill, you need to know only the number of premium channels to which the user has subscribed.
The output is the account number of the user and the billing amount.
For business customers, you must prompt users to enter the number of basic service connections and the number of premium channels. And store these inputs in variables number of basic service connections and number of premium channels.
To compute the business bill, you need to know the number of basic service connections and the number of premium channels to which the user subscribes. If the number of basic service connections is less than or equal to 10, the cost of the basic service connections is fixed.
If the number of basic service connections exceeds 10, you must add the cost for each connection over 10.
The output in this case is also the account number of the user and the billing amount.
If the customer code is incorrect or unacceptable, the program should output an error message.
The program is now ready and you can use it to print the user's account number and the amount due.
A sample run of the program looks like this. The program can be used to compute the bill for all types of customers.
image7.png
image8.png
image9.png
image10.png
image11.png
image12.png
image13.png
image14.png
image15.png
image16.png
image17.png
image18.png
image19.png
image20.png
image21.png
image1.png
image2.png
image3.png
image4.png
image5.png
image6.png
Collepals.com Plagiarism Free Papers
Are you looking for custom essay writing service or even dissertation writing services? Just request for our write my paper service, and we'll match you with the best essay writer in your subject! With an exceptional team of professional academic experts in a wide range of subjects, we can guarantee you an unrivaled quality of custom-written papers.
Get ZERO PLAGIARISM, HUMAN WRITTEN ESSAYS
Why Hire Collepals.com writers to do your paper?
Quality- We are experienced and have access to ample research materials.
We write plagiarism Free Content
Confidential- We never share or sell your personal information to third parties.
Support-Chat with us today! We are always waiting to answer all your questions.
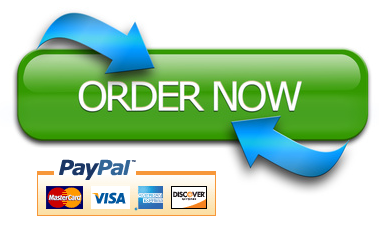