This assignment is an exercise in creating a “wrapper” class. A wrapper class is implemented by using a composition relationship and exporting only those functionalities required by the design
Introduction
The Object Oriented software model provides for both inheritance and composition
techniques for program design. Each has its advantages and disadvantages.
Assignment Description
This assignment is an exercise in creating a “wrapper” class. A wrapper class is
implemented by using a composition relationship and exporting only those functionalities
required by the design. In contrast, with inheritance all public members of the base class
are exported for use. Hence the composition relationship provides the means to customize
the behavior of the class object, and at the same time leverage the capabilities of the
already existing contained class.
In particular, this assignment creates a wrapper around the deque template class. Rather
than generalizing, which is the way we create reusable code, we will specialize and create
a list class containing strings. In practice storing and manipulating strings dominates
much of what is done by applications.
Program Requirements
1. The student will create the implementation for a class called SList. The interface for
the SList class will be provided with all applicable method declarations.
2. The student will create the implementation for the associated enumerator called
SListEnum, which is also declared in the file SList.h.
3. Each of the methods for both class and enumerator will be implemented using
“inline” functions. These inline functions will be included in the SList.h file.
4. The student will create a test driver to test each of the methods defined in the class.
The test driver file will be called TestSList.cpp.
5. The student will submit the source file for the test driver, TestSList.cpp, and the
SList.h file.
6. The student will “zip” only the source files for submission. The name of the zip file
will be “program1_studentlastname.zip”.
#ifndef SLIST_H_
#define SLIST_H_
#include
#include
class SListEnum;
class SList
{
public:
SList();
SList(const char* filename);
SList(const SList& L);
~SList();
const char* Add (const char*);
const char* Add (const std::string&);
SList& Move (SList& L,bool append=false);
void Sort (bool nodup=false); void Unique (); void Empty ();
SList* Split (const char* ,const char* delimiter);
const char* Join (std::string&,const char* delimiter); const char* Has (const char*);
void Read (const char* filename);
void Write (const char* filename);
bool operator==(SList&);
bool operator!=(SList&); SList& operator= (SList&);
SList& operator<<(const char* item);
friend class SListEnum;
const int count;
private: std::deque strlist;
}; //End of class SList
class SListEnum { public:
SListEnum (); SListEnum (SList& L);
SListEnum (SList* L);
~SListEnum();
void operator=(const SList& L);
void operator=(const SList* L);
void operator=(const SListEnum&);
bool hasMore();
const char* getNext();
private:
};
// IMPLEMENTATION GOES HERE
#endif
Collepals.com Plagiarism Free Papers
Are you looking for custom essay writing service or even dissertation writing services? Just request for our write my paper service, and we'll match you with the best essay writer in your subject! With an exceptional team of professional academic experts in a wide range of subjects, we can guarantee you an unrivaled quality of custom-written papers.
Get ZERO PLAGIARISM, HUMAN WRITTEN ESSAYS
Why Hire Collepals.com writers to do your paper?
Quality- We are experienced and have access to ample research materials.
We write plagiarism Free Content
Confidential- We never share or sell your personal information to third parties.
Support-Chat with us today! We are always waiting to answer all your questions.
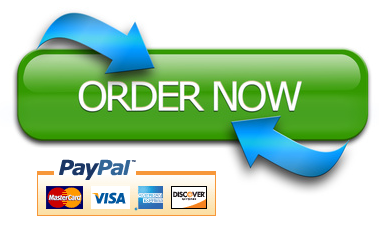