Create a Windows Forms Application With Basic Controls and Add an Employee Class This part of the project, you will create a Windows Forms application with an Employee class. Read the
Create a Windows Forms Application With Basic Controls and Add an Employee Class
This part of the project, you will create a Windows Forms application with an Employee class. Read the Project Guide provided and the project video link for instructions and details to help guide you through your efforts.
Project Files
Course Project DeVry University College of Engineering and Information Sciences
Screenshot of program running:
Form code (only the code for the form, not program.cs):
,
Course Project DeVry University College of Engineering and Information Sciences
Course Number: CEIS247
Background
This course project is designed to help you practice using your skills. Each week, you will learn important concepts. Then, you will apply these concepts to build an amazing course project over the next seven weeks. What’s more, this project will have a Graphical User Interface (GUI) instead of using the console. This course project has two parts. For the first couple of weeks, you will be create a basic GUI and learn how to work with GUI controls while practicing basic programming concepts. After that, you will create an object oriented project.
You have been hired as a consultant to create a basic Payroll System for a small business. The business wants to keep track of basic employee information and print paychecks for the employees every two weeks. Your client has some basic functionality in mind, such as the ability to add and remove employees, display basic employee information, and print paychecks. Maintenance is key! You realize that Object-Oriented programming will provide the most efficient application that is easy to maintain and update as needed. Let’s get started!
Week 5: Create a Windows forms application with basic controls and add an Employee Class
Objectives
1. To start the course project
2. Design and create two Windows forms with GUI controls
3. Read from and update GUI control variables
Introduction
Steps
1. Start Visual Studio. You will see a Start Page that looks something like this. Read through it, and then click the “Create a new project” tile near the bottom, right corner.
2. Create a new Windows Forms App (.NET Framework) – C# version. If you do not see Windows Forms App (.NET Framework), simply type “Windows Forms App” in the search box and choose the C# version. Then, hit the Next button.
3. When you configure your new project, call it “Lastname_CourseProject_part2”. Make sure you change the location to somewhere that you will find it easily! Then, click the Create button.
4. Make a new form. You should be presented with a blank form like the one below. You are now ready to start designing your form! Currently it is called Form1, which is both boring and poor programming practice. A form, as well as forms controls, should have meaningful names, just like all other variables.
Rename the form’s file in the Solution Explorer to MainForm. Then, add four Buttons and a ListBox to your form. Feel free to play with the Font, BackColor, or any other property! Have fun! The form above was set to a font size of 20. Feel free to do the same so your form looks like the one below.
Object |
Name |
Text |
Form |
MainForm |
Payroll System |
Button |
AddButton |
Add |
Button |
RemoveButton |
Remove |
Button |
DisplayButton |
Display |
Button |
PrintPaychecksButton |
Print Paychecks |
ListBox |
EmployeesListBox |
n/a |
Your main form should look something like this. If you hit the green Start arrow, your application will run! Congratulations, you have created your first form! Click on the little red square to stop the program execution and go back to the editor.
When we click the buttons, nothing happens. We have not “activated” the buttons yet. We need to add the code for each button to execute. When you double-click on a button, Visual Studio will create the Event Handler and the method to go with the event. For example, when you double-click on the Add button, Visual Studio will write this line to handle the event:
this.AddButton.Click += new System.EventHandler(this.AddButton_Click);
When a user clicks the AddButton, C# will run the “AddButton_Click” method. I highlighted the event and the method for clarity. In other words, the code that you put into the AddButton_Click will be executed when the user clicks the AddButton!
When the user clicks the AddButton, simply add a “New Employee” string to the employees listbox. When the user clicks the RemoveButton, get the selected item number (index) and remove the item at that item number. Check and make sure something is selected! When the user clicks the DisplayButton or the PrintPaychecksButton, show a message so the user knows that the button code has not been completed. When you finish, the code should look something like this:
using System;
using System.Windows.Forms;
namespace LastName_CourseProject_part2
{
public partial class MainForm : Form
{
public MainForm()
{
InitializeComponent();
}
private void AddButton_Click(object sender, EventArgs e)
{
// add item to the employee listbox
EmployeesListBox.Items.Add("New Employee");
}
private void RemoveButton_Click(object sender, EventArgs e)
{
// remove the selected item from the employee listbox
int itemNumber = EmployeesListBox.SelectedIndex;
if(itemNumber > -1 )
{
EmployeesListBox.Items.RemoveAt(itemNumber);
}
else
{
MessageBox.Show("Please select employee to remove.");
}
}
private void DisplayButton_Click(object sender, EventArgs e)
{
MessageBox.Show("Displaying all employees…");
}
private void PrintPaychecksButton_Click(object sender, EventArgs e)
{
MessageBox.Show("Printing paychecks for all employees…");
}
}
}
Deliverables Week 5
· Use the Word document template and put your information at the top. Take a screenshot while your application is running. Paste the screenshot to a Word document below your information. Finally, copy-paste your Form code (only the code for the form, not program.cs) to the Word document below the screenshot. Paste a screenshot of your Employee class. Save and close your Word document. Submit the Word document for your first Course Project deliverable.
Employee <<Stereotype>> parameter – firstName : string + ToString( ) : string – lastName : string – ssn : string – hireDate : DateTime + CalculatePay( ) : double
,
Lavf58.12.100
Collepals.com Plagiarism Free Papers
Are you looking for custom essay writing service or even dissertation writing services? Just request for our write my paper service, and we'll match you with the best essay writer in your subject! With an exceptional team of professional academic experts in a wide range of subjects, we can guarantee you an unrivaled quality of custom-written papers.
Get ZERO PLAGIARISM, HUMAN WRITTEN ESSAYS
Why Hire Collepals.com writers to do your paper?
Quality- We are experienced and have access to ample research materials.
We write plagiarism Free Content
Confidential- We never share or sell your personal information to third parties.
Support-Chat with us today! We are always waiting to answer all your questions.
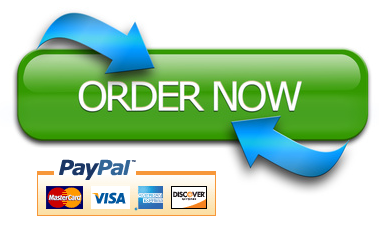