I’m looking for someone to help me with this task. It will run in Python IDLE, and all you have to do is read the file and follow the instruction
I'm looking for someone to help me with this task. It will run in Python IDLE, and all you have to do is read the file and follow the instructions. It MUST be implemented in the same way as it is in the file, and functions must run independently, as it will be marked if the functions are running or not, and it must be implemented in the correct structure.
from __future__ import annotations from typing import Optional from a2_support import UserInterface, TextInterface from constants import * # Replace these <strings> with your name, student number and email address. __author__ = "<Your Name>, <Your Student Number>" __email__ = "<Your Student Email>" # Before submission, update this tag to reflect the latest version of the # that you implemented, as per the blackboard changelog. __version__ = 1.0 # Uncomment this function when you have completed the Level class and are ready # to attempt the Model class. # def load_game(filename: str) -> list['Level']: # """ Reads a game file and creates a list of all the levels in order. # Parameters: # filename: The path to the game file # Returns: # A list of all Level instances to play in the game # """ # levels = [] # with open(filename, 'r') as file: # for line in file: # line = line.strip() # if line.startswith('Maze'): # _, _, dimensions = line[5:].partition(' – ') # dimensions = [int(item) for item in dimensions.split()] # levels.append(Level(dimensions)) # elif len(line) > 0 and len(levels) > 0: # levels[-1].add_row(line) # return levels # Write your classes here def main(): # Write your code here pass if __name__ == '__main__': main()
,
from constants import PLAYER class UserInterface: """ Abstract class providing an interface for any MazeRunner View class. """ def draw( self, maze: 'Maze', items: dict[tuple[int, int], 'Item'], player_position: tuple[int, int], inventory: 'Inventory', player_stats: tuple[int, int, int] ) -> None: """ Draws the current game state. Parameters: maze: The current Maze instance items: The items on the maze player_position: The position of the player inventory: The player's current inventory player_stats: The (HP, hunger, thirst) of the player """ self._draw_level(maze, items, player_position) self._draw_inventory(inventory) self._draw_player_stats(player_stats) def _draw_inventory(self, inventory: 'Inventory') -> None: """ Draws the inventory information. Implemented in subclasses. Parameters: inventory: The player's current inventory """ raise NotImplementedError def _draw_player_stats(self, player_stats: tuple[int, int, int]) -> None: """ Draws the players stats. Implemented in subclasses. Parameters: player_stats: The player's current (HP, hunger, thirst) """ raise NotImplementedError def _draw_level( self, maze: 'Maze', items: dict[tuple[int, int], 'Item'], player_position: tuple[int, int] ) -> None: """ Draws the maze and all its items. Implemented in subclasses. Parameters: maze: The current maze for the level items: Maps locations to the items currently at those locations player_position: The current position of the player """ raise NotImplementedError class TextInterface(UserInterface): """ A MazeRunner interface that uses ascii to present information. """ def _draw_level( self, maze: 'Maze', items: dict[tuple[int, int], 'Item'], player_position: tuple[int, int] ) -> None: num_rows, num_cols = maze.get_dimensions() for row in range(num_rows): row_str = '' for col in range(num_cols): if (row, col) == player_position: row_str += PLAYER elif (row, col) in items: row_str += items.get((row, col)).get_id() else: row_str += maze.get_tile((row, col)).get_id() print(row_str) def _draw_inventory(self, inventory: 'Inventory') -> None: text = str(inventory) if inventory.get_items() != {} else 'Empty' print('—————nInventoryn' + text + 'n' + '—————') def _draw_player_stats(self, player_stats: tuple[int, int, int]) -> None: hp, hunger, thirst = player_stats print(f'HP: {hp}nhunger: {hunger}nthirst: {thirst}')
,
LAVA = 'L' WALL = '#' EMPTY = ' ' DOOR = 'D' PLAYER = 'P' ITEM = 'I' FOOD = 'F' DYNAMIC_ENTITY = 'DE' ABSTRACT_TILE = 'AT' COIN = 'C' POTION = 'M' HONEY = 'H' APPLE = 'A' WATER = 'W' APPLE_AMOUNT = -1 HONEY_AMOUNT = -5 WATER_AMOUNT = -5 POTION_AMOUNT = 20 UP = 'w' DOWN = 's' LEFT = 'a' RIGHT = 'd' MOVE_DELTAS = { UP: (-1, 0), DOWN: (1, 0), LEFT: (0, -1), RIGHT: (0, 1), } MAX_HEALTH = 100 MAX_HUNGER = 10 MAX_THIRST = 10 LAVA_DAMAGE = 5 WIN_MESSAGE = 'Congratulations! You have finished all levels and won the game!' LOSS_MESSAGE = 'You lose :(' ITEM_UNAVAILABLE_MESSAGE = 'nYou don't have any of that item!n'
,
Maze 1 – 5 5 ##### # C D # C # P C # ##### Maze 2 – 7 8 ######## P # ###### # # # # ###### # # ######D#
,
Maze 1 – 5 5 ##### # C D # C # P C # ##### Maze 2 – 7 8 ######## P M H # ###### # #LL M A# #L###### #LLL # ######D#
,
Maze 1 – 5 5 ##### # C D # C # P CC# ##### Maze 2 – 20 19 ################### # # # #M # P ## # # ## # ### # # #M C#C#C# M# #########C#C#C# ### # A #C#CCC# H# # ##### ####### # # # #CCC# # # # # ###CCC##### ### # # ##### # C# # # # H #####C# # #### ###### #C# # # # # # #C# ### # ## # # ## ### # M# # # # D #C######## ## ### # #C# # # # #C# ######### ##### #C# A CCCC# ###################
Collepals.com Plagiarism Free Papers
Are you looking for custom essay writing service or even dissertation writing services? Just request for our write my paper service, and we'll match you with the best essay writer in your subject! With an exceptional team of professional academic experts in a wide range of subjects, we can guarantee you an unrivaled quality of custom-written papers.
Get ZERO PLAGIARISM, HUMAN WRITTEN ESSAYS
Why Hire Collepals.com writers to do your paper?
Quality- We are experienced and have access to ample research materials.
We write plagiarism Free Content
Confidential- We never share or sell your personal information to third parties.
Support-Chat with us today! We are always waiting to answer all your questions.
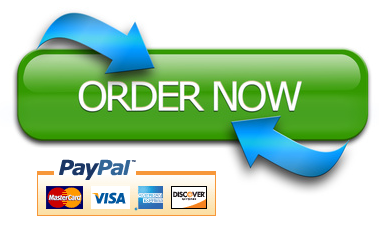