Validating input from a form and Looping through Songs Instructions are attached Its continuation of what you startedCEIS209ProjectD
Do week 2;
Week 2: Validating input from a form and Looping through Songs
Instructions are attached
Its continuation of what you started
Course Project DeVry University College of Engineering and Information Sciences
Screenshot of program running:
Form code (only the code for the form, not program.cs):
,
Course Project DeVry University College of Engineering and Information Sciences
Course Number: CEIS209
Background
Part 1: Scenario
Lucky you! Your manager has put you in charge of the lyrics videos for the monthly lip synch contest. Each contestant “sings” along with a song of their choice, prompted by the lyrics in the video. Essentially, there is a long list of songs, along with associated URLs and other information. Your manager has some basic functionality in mind, such as the ability to add and remove songs, as well as create playlists and show the videos. The application must run fast, and be updated with new features over time. Maintenance is key!
NOTE: For this project, we strongly suggest keeping a Notepad or MS Word document handy, containing the names of a few songs and associated video URLs. The URLs can be local video files on your hard drive, or videos on web-based sites such as YouTube.com.
Week 2: Validating input from a form and Looping through Songs
Objectives
1. Use IF statements to determine if textboxes are empty
2. Use MessageBox.Show to display error/success messages
Introduction
This week, you will add three songs, and display each one in your outputText textbox. However, you will also be checking that data entered into the form is formatted correctly (e.g., no blank form fields).
When the user clicks the “Add Song” button after each one, and they made an error, pop up an error message and do not add the song. If they did not make an error, then pop up a message “Song added!” and display the song information in the outputText textbox. If you consider WHERE to put your checks for empty textboxes, ask yourself, “At what point should the checking happen?” The answer is “when the user clicks ‘Add’!” Therefore, the changes you will make this week are inside the AddButton_Click event handler that you wrote last week.
In addition to error validation, you will add and display song data more efficiently with a ListBox. So, instead of just having the AddButton_Click event handler write to your outputText, you will have it write to the listbox instead. Listboxes are particularly useful because you can have a user select something from a list box.
Steps
1. There are two important Windows Forms methods that you will be using this week:
a. To test if a string (such as the Text field of a textbox) is empty or null, use the string.IsNullOrEmpty(string) method
b. To pop up a MessageBox with a message and an OK button, use MessageBox.Show(message).
2. Open your course project solution from last week. It should look something like the screenshot below.
3. Revise the programming logic in the AddButton_Click event handler. Double-click on the “Add Song” button to bring up the code for the event handler. It should be similar to the code below.
private void AddButton_Click(object sender, EventArgs e)
{
StringBuilder sb = new StringBuilder(outputText.Text);
string nl = "rn";
// Build the output text
sb.Append(titleText.Text);
sb.Append(nl);
sb.Append(artistText.Text);
sb.Append(nl);
sb.Append(genreText.Text);
sb.Append(nl);
sb.Append(yearText.Text);
sb.Append(nl);
sb.Append(urlText.Text);
sb.Append(nl);
outputText.Text = sb.ToString();
}
4. Looking at the code, where do you want the error checking to occur? The flowchart below shows the logical steps. First, you check to see if the title is empty. If it is, display an error message (in a MessageBox) and exit AddButton_Click. If not, then check the artist, and so on.
5. Edit the code in AddButton_Click to reflect the logic in the flowchart. The first two checks are done for you below. Update your AddButton_Click method with the new code, and then add the code for the genre, year, and URL textboxes.
6. Take a screenshot showing an error message and paste it in the report.
7. Add a new ListBox. On your form, shift your outputText down toward the bottom of the form, and add a ListBox (NOT a ListView) above it.
8. Change the properties of the listbox as follows:
a. Give it the variable name songList (it’s under “Design” in the properties)
b. HorizontalScrollbar = true
c. Multicolumn = false
d. ScrollAlwaysVisible = true
9. Add another button to your form. Call it allSongsButton, and set the text on the button to “Show all songs.” The purpose of this button will be to take all of the song titles in your new songList listbox and display them in the outputText textbox. Your forms should now be similar to the one pictured below.
Notice that I also added labels “Song List” and “Details.” Don’t hesitate to be creative with the design!
10. Update the AddButton_Click event handler to work with the ListBox. At this point, keep the StringBuilder code that displays the song information to the outputText textbox. You also want to add an item (a song title) to the songList listbox. Make sure that you add it AFTER you know that there are no blank textboxes.
To add an item to a listbox, you use songList.Items.Add(titleText.Text);
11. Display the contents of the songList. This is the purpose of the allSongs button – to read each item in the songList and display it in the outputText. For now you can display only the song titles. Later in the project, you will display the artist, genre, year, and URL as well.
Double-click on the “Show All Songs” button to bring up its event handler. To display all of the songs, you should loop through the items in the songList, and append each title onto a string. As you did with AddButton_Click, you will need a StringBuilder. It is also a good idea to clear the contents of the outputText textbox, so that you don’t have text from previous operations there.
On Your Own
Research tab indexes and ensure that when you tab through each of the text boxes, it follows a reasonable order. Then test your program by running it and tabbing through all of the text boxes.
Deliverables Week 2
· Use the word document template and put your information at the top, including your name, course number, and current date. Take a screenshot while your application is running, showing your pop-up MessageBoxes, both for an error and for a successful add. Paste the screenshots into a Word document below your information. Then paste the body of your AddButton_Click event handler code. Also, show the result of adding at least three songs, then clicking on “Show All Songs.” Paste the screenshot into a Word document below your information, followed by your AllSongsButton_Click code.
Collepals.com Plagiarism Free Papers
Are you looking for custom essay writing service or even dissertation writing services? Just request for our write my paper service, and we'll match you with the best essay writer in your subject! With an exceptional team of professional academic experts in a wide range of subjects, we can guarantee you an unrivaled quality of custom-written papers.
Get ZERO PLAGIARISM, HUMAN WRITTEN ESSAYS
Why Hire Collepals.com writers to do your paper?
Quality- We are experienced and have access to ample research materials.
We write plagiarism Free Content
Confidential- We never share or sell your personal information to third parties.
Support-Chat with us today! We are always waiting to answer all your questions.
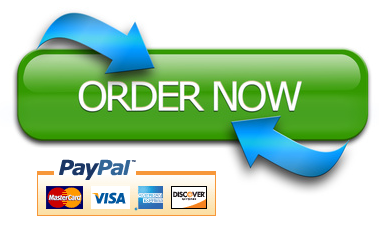