Check The folder saying Lab 1 that where you will find the questions. Rest are help Write a program that inputs (use JOptionPane.sh
Check The folder saying Lab 1 that where you will find the questions. Rest are help
Write a program that inputs (use JOptionPane.showInputDialog method) four numbers and graphs them as a pie chart. Use class Arc2D.Double and method fill of class Graphics2D to perform the drawing. Draw each piece of the pie in a separate color.
Given program (Lab_1_2_start_Up) is an application that allows the user to select a shape from a JComboBox and draws it 20 times with random locations and dimensions in method paintComponent. The first item in the JComboBox should be the default shape that is displayed the first time paintComponent is called. (Screenshot 1 & 2)
Lab_1_1_Help/Shapes.java
Lab_1_1_Help/Shapes.java
// Lab 1 Question 1 help
import javax . swing . * ;
public class Shapes
{
// execute application
public static void main ( String [] args )
{
int length ;
int width ;
// create frame for ShapesJPanel
JFrame frame = new JFrame ( "Drawing 2D shapes" );
frame . setDefaultCloseOperation ( JFrame . EXIT_ON_CLOSE );
// input the length and width
length = Integer . parseInt ( JOptionPane . showInputDialog (
frame , "Please enter the length:" ));
width = Integer . parseInt ( JOptionPane . showInputDialog (
frame , "Please enter the width:" ));
// create ShapesJPanel
ShapesJPanel shapesJPanel = new ShapesJPanel ( length , width );
frame . add ( shapesJPanel );
frame . setSize ( 425 , 200 );
frame . setVisible ( true );
}
} // end class Shapes
Lab_1_1_Help/ShapesJPanel.java
Lab_1_1_Help/ShapesJPanel.java
// Lab 1 Question 1 help
import java . awt . * ;
import java . awt . event . ActionEvent ;
import java . awt . event . ActionListener ;
import java . awt . geom . Rectangle2D ;
import javax . swing . * ;
public class ShapesJPanel extends JPanel
{
private int length ;
private int width ;
private JButton exitJButton ;
//constructor to receive inputs
public ShapesJPanel ( int length , int width ){
this . length = length ;
this . width = width ;
exitJButton = new JButton ( "Exit" );
exitJButton . addActionListener (
new ActionListener () {
@ Override
public void actionPerformed ( ActionEvent e ) {
int result = JOptionPane . showConfirmDialog ( null , "Are you Sure?" );
System . out . println ( result );
if ( result == 0 ){
JOptionPane . showMessageDialog ( null , "Thank you for using this program" , "Bye!" , JOptionPane . PLAIN_MESSAGE );
//dispose();
System . exit ( 0 );}
}
}
);
this . setLayout ( new BorderLayout ());
this . add ( exitJButton , BorderLayout . SOUTH );
}
// draw shapes with Java 2D API
@ Override
public void paintComponent ( Graphics g )
{
super . paintComponent ( g );
Graphics2D g2d = ( Graphics2D ) g ; // cast g to Graphics2D
// draw 2D rectangle in red
g2d . setPaint ( Color . RED );
g2d . setStroke ( new BasicStroke ( 10.0f ));
g2d . draw ( new Rectangle2D . Double ( 80 , 30 , width , length ));
}
} // end class ShapesJPanel
,
Lab_1_2_StartUp/SelectShape.java
Lab_1_2_StartUp/SelectShape.java
// Lab 1 Question 2 StartUp file
import javax . swing . JFrame ;
public class SelectShape
{
public static void main ( String args [])
{
SelectShapeJFrame selectShapeJFrame = new SelectShapeJFrame ();
selectShapeJFrame . setDefaultCloseOperation ( JFrame . EXIT_ON_CLOSE );
selectShapeJFrame . setSize ( 420 , 470 ); // set frame size
selectShapeJFrame . setVisible ( true ); // display frame
}
} // end class SelectShape
/**************************************************************************
* (C) Copyright 1992-2018 by Deitel & Associates, Inc. and *
* Prentice Hall. All Rights Reserved. *
* *
* DISCLAIMER: The authors and publisher of this book have used their *
* best efforts in preparing the book. These efforts include the *
* development, research, and testing of the theories and programs *
* to determine their effectiveness. The authors and publisher make *
* no warranty of any kind, expressed or implied, with regard to these *
* programs or to the documentation contained in these books. The authors *
* and publisher shall not be liable in any event for incidental or *
* consequential damages in connection with, or arising out of, the *
* furnishing, performance, or use of these programs. *
*************************************************************************/
Lab_1_2_StartUp/SelectShapeJFrame.java
Lab_1_2_StartUp/SelectShapeJFrame.java
// Lab 1 Question 2 StartUp file
// Draw a shape 20 times in random positions
import java . awt . BorderLayout ;
import java . awt . event . ItemListener ;
import java . awt . event . ItemEvent ;
import javax . swing . JComboBox ;
import javax . swing . JFrame ;
public class SelectShapeJFrame extends JFrame
{
private ShapeOption shapeOptions [] = { ShapeOption . OVAL , ShapeOption . RECTANGLE };
private ShapeOption shape = ShapeOption . OVAL ;
private JComboBox < ShapeOption > choiceJComboBox ;
private SelectShapeJPanel selectShapeJPanel ;
public SelectShapeJFrame ()
{
super ( "Selecting Shapes" );
selectShapeJPanel = new SelectShapeJPanel ();
choiceJComboBox = new JComboBox < ShapeOption > ( shapeOptions );
choiceJComboBox . addItemListener (
new ItemListener () // anonymous inner class
{
public void itemStateChanged ( ItemEvent e )
{
selectShapeJPanel . setShape (
( ShapeOption ) choiceJComboBox . getSelectedItem ());
repaint ();
}
}
);
add ( selectShapeJPanel , BorderLayout . CENTER );
add ( choiceJComboBox , BorderLayout . SOUTH );
}
} // end class SelectShapeJFrame
Lab_1_2_StartUp/SelectShapeJPanel.java
Lab_1_2_StartUp/SelectShapeJPanel.java
// Lab 1 Question 2 StartUp file
// Draw a shape 20 times in random positions
import java . awt . Color ;
import java . awt . Graphics ;
import java . util . Random ;
import javax . swing . JPanel ;
public class SelectShapeJPanel extends JPanel
{
private final int SIZE = 400 ;
private ShapeOption shape = ShapeOption . OVAL ;
// draw the new shape in random locations 20 times
public void paintComponent ( Graphics g )
{
super . paintComponent ( g );
Random random = new Random (); // get random-number generator
for ( int count = 1 ; count <= 20 ; count ++ )
{
// add 10 and 25 to prevent drawing over edge
int x = ( int ) ( random . nextFloat () * SIZE ) + 10 ;
int y = ( int ) ( random . nextFloat () * SIZE ) + 25 ;
int width = ( int ) ( random . nextFloat () * ( SIZE - x ));
int height = ( int ) ( random . nextFloat () * ( SIZE - y ));
g . setColor ( Color . BLUE );
// draw the appropriate shape
switch ( shape )
{
case OVAL :
g . drawOval ( x , y , width , height );
break ;
case RECTANGLE :
g . drawRect ( x , y , width , height );
break ;
}
}
}
// set new shape
public void setShape ( ShapeOption preference )
{
shape = preference ;
}
} // end class SelectShapeJPanel
Lab_1_2_StartUp/ShapeOption.java
Lab_1_2_StartUp/ShapeOption.java
// Lab 1 Question 2 StartUp file
// Defines an enum type for the program's shape options.
public enum ShapeOption
{
// declare contents of enum type
OVAL ( 1 ),</s
Collepals.com Plagiarism Free Papers
Are you looking for custom essay writing service or even dissertation writing services? Just request for our write my paper service, and we'll match you with the best essay writer in your subject! With an exceptional team of professional academic experts in a wide range of subjects, we can guarantee you an unrivaled quality of custom-written papers.
Get ZERO PLAGIARISM, HUMAN WRITTEN ESSAYS
Why Hire Collepals.com writers to do your paper?
Quality- We are experienced and have access to ample research materials.
We write plagiarism Free Content
Confidential- We never share or sell your personal information to third parties.
Support-Chat with us today! We are always waiting to answer all your questions.
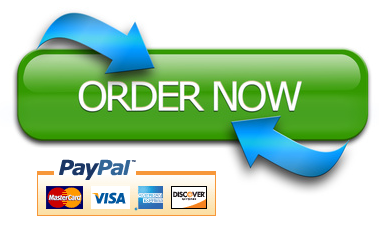
All Rights Reserved Terms and Conditions
College pals.com Privacy Policy 2010-2018