Implement stack using STL by extending Bag Interface class. Also use exception handling (for error handling) and file hand
Implement stack using STL by extending Bag Interface class. Also use exception handling (for error handling) and file handling (to read in the inputs)
DS/.DS_Store
__MACOSX/DS/._.DS_Store
DS/ArrayBagTest.cpp
#include <iostream> // For cout and cin #include <string> // For string objects #include "ArrayBag.h"// For ADT bag #include "ArrayBag.cpp" #include "StackSTL.h" #include "StackSTL.cpp" #include <stack> #include "BagInterface.h" using namespace std; int main() { /*ArrayBag<char> bags; bags.add('a'); bags.print(); bags.add('b'); bags.print(); cout<<(bags.contains('a') ? "YES" : "NO")<<endl; cout<<bags.getCurrentSize()<<endl; bags.print(); bags.remove('a'); bags.print(); cout<<(bags.contains('a') ? "YES" : "NO")<<endl; cout<<bags.getCurrentSize()<<endl; for(char x: bags.toVector()) cout<<x<< " "; cout<<endl;*/ cout<<"Testing Stack STL Implementation"<<endl; // BagInterface<char> *bag = stack<char>(); stack<char>(); bag->add('a'); //bag->print(); return 0; } // end main
__MACOSX/DS/._ArrayBagTest.cpp
DS/BagInterface.h
#ifndef BAG_INTERFACE_H #define BAG_INTERFACE_H #include<iostream> #include<vector> #include<algorithm> using namespace std; template <class ItemType> class BagInterface { public: ~BagInterface() {} virtual int getCurrentSize(void) const = 0; virtual bool isEmpty(void) const = 0; virtual bool add(const ItemType& item) = 0; virtual bool remove(const ItemType& item) = 0; virtual void clear(void) = 0; void print() const; virtual int getFrequencyOf(const ItemType& item) const = 0; virtual bool contains(const ItemType& item) const = 0; virtual vector<ItemType> toVector(void) const = 0; }; #endif
__MACOSX/DS/._BagInterface.h
DS/ArrayBag.cpp
#include <vector> #include "ArrayBag.h" template <class ItemType> ArrayBag<ItemType>::ArrayBag():itemCount(0),maxItems(DEFAULT_CAPACITY) { } // default constructor template <class ItemType> int ArrayBag <ItemType>::getCurrentSize() const { return itemCount; } // end getCurrentSize template <class ItemType> bool ArrayBag <ItemType>::isEmpty() const { return itemCount == 0; } // end isEmpty template <class ItemType> bool ArrayBag<ItemType>::add(const ItemType& newEntry) { bool hasRoomToAdd(itemCount < maxItems); if (hasRoomToAdd) { items[itemCount] = newEntry; itemCount++; } return hasRoomToAdd; } // end add function template <class ItemType> bool ArrayBag<ItemType>::remove(const ItemType& anEntry) { int locatedIndex = getIndexOf(anEntry); bool canRemoveItem = !isEmpty() && (locatedIndex > -1); if (canRemoveItem) { itemCount–; items[locatedIndex] = items[itemCount]; } return canRemoveItem; } // end remove template <class ItemType> void ArrayBag<ItemType>::clear() { itemCount = 0; } // end clear template <class ItemType> bool ArrayBag<ItemType>::contains(const ItemType& anEntry) const { int curIndex(0); while (curIndex < itemCount) { if (items[curIndex] == anEntry) { return true; } curIndex++; } return false; } // end contains template <class ItemType> int ArrayBag<ItemType>::getFrequencyOf(const ItemType& anEntry) const { int frequency(0); int curIndex(0); while (curIndex < itemCount) { if (items[curIndex] == anEntry) { frequency++; } curIndex++; } return frequency; } // end getFrequency template <class ItemType> vector<ItemType> ArrayBag<ItemType>::toVector() const { vector<ItemType> bagContents; for (int i=0; i < itemCount; i++) bagContents.push_back(items[i]); return bagContents; } // end vector template <class ItemType> void ArrayBag<ItemType>::print() const { for(int i = 0; i < itemCount; i++) cout << items[i] << " "; cout << endl; } // end print template <class ItemType> int ArrayBag<ItemType>::getIndexOf(const ItemType& target) const { bool found = false; int result = -1; int searchIndex = 0; while(!found && (searchIndex < itemCount)) { if(items[searchIndex] == target) { found = true; result = searchIndex; } else { searchIndex++; } } return result; } // end getIndexOf
__MACOSX/DS/._ArrayBag.cpp
DS/ArrayBag.h
#ifndef ARRAY_BAG_H #define ARRAY_BAG_H #include <vector> #include "BagInterface.h" template <class ItemType> class ArrayBag : public BagInterface<ItemType> { public: ArrayBag(); ~ArrayBag(){}; int getCurrentSize() const; bool isEmpty() const; bool add(const ItemType& item); bool remove(const ItemType& item); bool contains(const ItemType& anEntry) const; void clear(); void print() const; int getFrequencyOf(const ItemType& item) const; vector<ItemType> toVector() const; private: static const int DEFAULT_CAPACITY = 6; ItemType items[DEFAULT_CAPACITY]; int itemCount; int maxItems; int getIndexOf(const ItemType& item) const; }; #endif
__MACOSX/DS/._ArrayBag.h
DS/StackSTL.h
#ifndef STACKSTL_H #define STACKSTL_H #include "BagInterface.h" #include <stack> template <class ItemType> class StackSTL : public BagInterface<ItemType> { public: StackSTL(); bool add(const ItemType& newEntry); /*bool remove(const ItemType& anEntry); virtual ~StackSTL() { } void print() const;*/ private: stack<ItemType> stackBag; }; #include "StackSTL.cpp" #endif
__MACOSX/DS/._StackSTL.h
DS/a.out
DS/StackSTL.cpp
#ifndef STACKSTL_CPP #define STACKSTL_CPP #include<iostream> #include<string> #include<cstddef> #include <stack> using namespace std; template<class ItemType> StackSTL<ItemType>::StackSTL(){} template <class ItemType > bool StackSTL<ItemType>::add(const ItemType & newEntry) { stackBag.push(newEntry); } //end push function /*template < class ItemType > bool StackSTL<ItemType>::remove(const ItemType & anEntry) { stackBag.pop(anEntry); } *///end pop #endif
__MACOSX/DS/._StackSTL.cpp
Collepals.com Plagiarism Free Papers
Are you looking for custom essay writing service or even dissertation writing services? Just request for our write my paper service, and we'll match you with the best essay writer in your subject! With an exceptional team of professional academic experts in a wide range of subjects, we can guarantee you an unrivaled quality of custom-written papers.
Get ZERO PLAGIARISM, HUMAN WRITTEN ESSAYS
Why Hire Collepals.com writers to do your paper?
Quality- We are experienced and have access to ample research materials.
We write plagiarism Free Content
Confidential- We never share or sell your personal information to third parties.
Support-Chat with us today! We are always waiting to answer all your questions.
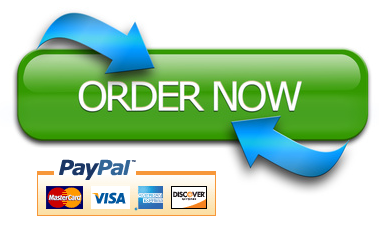